Random Forest
A random forest classifier is a machine learning algorithm that is used for classification tasks. It is an ensemble method that involves training multiple decision tree classifiers on subsets of the data and then averaging the predictions of all the individual classifiers to make a final prediction.
Random forest classifiers are often considered to be among the most accurate and robust machine learning algorithms, and they are widely used in a variety of applications, including image and text classification, fraud detection, and predictive maintenance.
A random forest classifier is a meta-estimator that fits a number of decision trees on various sub-samples of datasets and uses average to improve the predictive accuracy of the model and controls over-fitting. The sub-sample size is always the same as the original input sample size but the samples are drawn with replacement.
Advantages: Reduction in over-fitting and random forest classifier is more accurate than decision trees in most cases.
Disadvantages: Slow real-time prediction, difficult to implement, and complex algorithm.
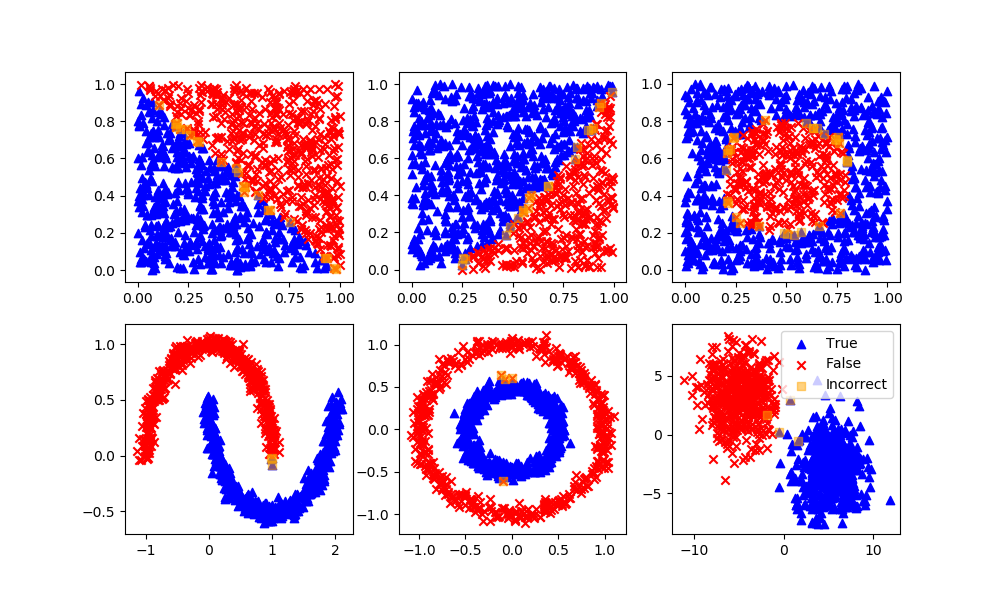
Random Forest Classifier in Python
Here is an example of how a random forest classifier might be implemented in Python using the scikit-learn library:
from sklearn.ensemble import RandomForestClassifier
# Create a random forest classifier
classifier = RandomForestClassifier(n_estimators=100)
# Train the classifier on the training data
classifier.fit(X_train, y_train)
# Use the classifier to make predictions on the test data
predictions = classifier.predict(X_test)
# Evaluate the performance of the classifier
accuracy = classifier.score(X_test, y_test)
In this code, the RandomForestClassifier class from the sklearn.ensemble library is used to create a random forest classifier. The classifier is then trained on a dataset of labeled training data (represented by the X_train and y_train variables) using the fit method. The classifier can then be used to make predictions on new data (represented by the X_test variable) using the predict method. Finally, the performance of the classifier can be evaluated by comparing its predictions to the true labels of the test data (represented by the y_test variable) using the score method. There are additional hyper-parameters (options) that influence the accuracy, memory requirements, and speed of training and prediction.
rfm = RandomForestClassifier(n_estimators=70,oob_score=True,n_jobs=1,\
random_state=101,max_features=None,min_samples_leaf=3)
rfm.fit(XA,yA)
yP = rfm.predict(XB)
OCR with Random Forest Classifier

Optical character recognition (OCR) is a technology that enables the recognition of text characters in digital images. This technology can be used to automatically convert scanned documents, pictures, or other digital images that contain text into machine-readable text.
Random forest is a type of machine learning algorithm that is commonly used in the field of OCR. This algorithm is an ensemble method that involves training multiple decision tree classifiers on subsets of the data and then averaging the predictions of all the individual classifiers to make a final prediction.
In the context of OCR, a random forest classifier can be trained to recognize text characters in images by learning from a large dataset of labeled images. The classifier is presented with many examples of each character, and uses these examples to learn the visual patterns that are associated with each character.
Once the classifier has been trained, it can be used to make predictions on new images. Given an image of a text character, the classifier will analyze the visual patterns in the image and make a prediction about which character is most likely to be present. Below is an example of how this might be implemented in Python using the scikit-learn library using a train/test split.
from sklearn.model_selection import train_test_split
import matplotlib.pyplot as plt
import numpy as np
from sklearn.ensemble import RandomForestClassifier
classifier = RandomForestClassifier(n_estimators=70,oob_score=True,n_jobs=1,\
random_state=101,max_features=None,min_samples_leaf=3)
# The digits dataset
digits = datasets.load_digits()
n_samples = len(digits.images)
data = digits.images.reshape((n_samples, -1))
# Split into train and test subsets (50% each)
X_train, X_test, y_train, y_test = train_test_split(
data, digits.target, test_size=0.5, shuffle=False)
# Learn the digits on the first half of the digits
classifier.fit(X_train, y_train)
# Test on second half of data
n = np.random.randint(int(n_samples/2),n_samples)
print('Predicted: ' + str(classifier.predict(digits.data[n:n+1])[0]))
# Show number
plt.imshow(digits.images[n], cmap=plt.cm.gray_r, interpolation='nearest')
plt.show()
MATLAB Live Script
✅ Knowledge Check
1. Which of the following is a primary advantage of the Random Forest classifier?
- Incorrect. One of the disadvantages of Random Forest is its slow real-time prediction. While it might be robust and accurate, it can be slower than some other algorithms due to the ensemble nature of combining multiple decision trees.
- Correct. Random Forest is an ensemble method that involves training multiple decision tree classifiers and averaging their predictions to improve predictive accuracy and control over-fitting.
- Incorrect. While Random Forest can be used for image data (such as in OCR), it is not exclusive to it. Random Forest can be used for a variety of classification tasks.
- Incorrect. Random Forest is known for its reduction in over-fitting, not under-fitting. This is due to its ensemble nature which tends to average out the biases of individual trees.
2. How does Random Forest differ from a simple decision tree?
- Incorrect. Typically, a Random Forest classifier is more accurate than a single decision tree. This is because it averages out the results of multiple decision trees, reducing variance and over-fitting.
- Incorrect. Random Forest can be used for both classification and regression tasks. It is not limited to just regression.
- Correct. One of the primary differences between Random Forest and a single decision tree is that Random Forest involves training multiple decision trees on sub-samples of the data. These sub-samples are often drawn with replacement.
- Incorrect. Random Forest is more complex because it's an ensemble method that combines the results of multiple decision trees. A single decision tree is simpler in comparison.
Return to Classification Overview