Smartphone Sensors
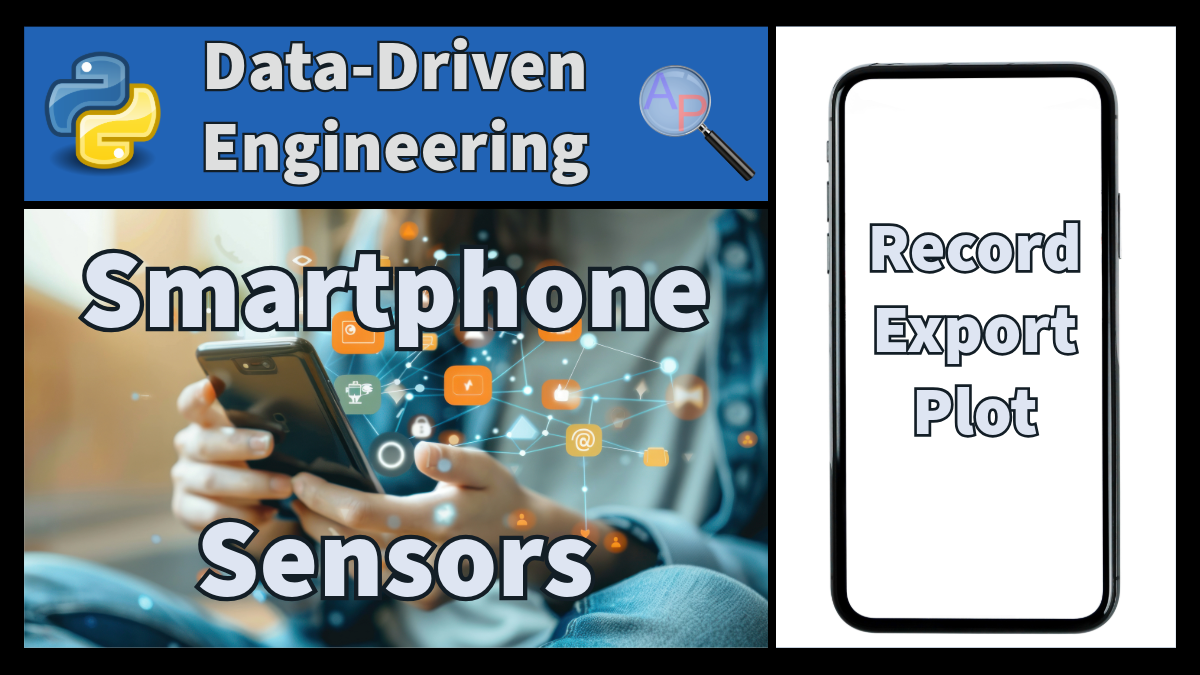
Modern smartphones come equipped with a wide array of sensors that enhance the user experience and enable various functionalities. These sensors fall into several categories: motion, environmental, positional, biometric, and proximity sensors.
Motion Sensors
Motion sensors like accelerometers and gyroscopes enable dynamic functions. The accelerometer detects changes in motion and orientation across three axes, allowing automatic screen rotation and step counting. The gyroscope offers more precise motion detection, essential for gaming and augmented reality applications.
Environmental Sensors
Environmental sensors include barometers, thermometers, and ambient light sensors. The barometer measures atmospheric pressure, helping determine altitude and enhance GPS accuracy. Thermometers monitor device temperature to prevent overheating, while ambient light sensors adjust screen brightness based on ambient light, improving visibility and conserving battery life.
Positional Sensors
Positional sensors, such as magnetometers and GPS modules, provide orientation and precise location data. The magnetometer functions as a digital compass, while the GPS module communicates with satellites to provide location data, essential for navigation and location-based services.
Biometric Sensors
Biometric sensors, including fingerprint scanners and facial recognition systems, offer secure authentication. Fingerprint scanners use capacitive or ultrasonic technology for secure access, while facial recognition uses infrared sensors and cameras for hands-free unlocking.
Proximity Sensors
Proximity sensors detect nearby objects without physical contact, often used to turn off the display during phone calls when held near the ear, preventing accidental touches.
Applications of Smartphone Sensors
- Navigation and Mapping - GPS and magnetometers enable precise location tracking and navigation.
- Health and Fitness - Accelerometers and biometric sensors track physical activity and health metrics.
- Gaming and Augmented Reality - Gyroscopes and accelerometers enhance interactive gaming experiences.
- Photography - Ambient light sensors and motion data help adjust camera settings and stabilize images.
- Security - Biometric sensors provide secure authentication.
- Environmental Monitoring - Barometers and thermometers measure atmospheric pressure and temperature, useful for weather forecasting.
Smartphones, through these sensors, have transformed into versatile tools capable of performing many tasks beyond traditional communication.
✅ Activity: Orientation
Objective: Use a smartphone to record orientation data (roll, pitch, and yaw) and then import and visualize this data in Python. Gain experience in data collection, CSV handling, and plotting in Python.
Install a Sensor Logger App
Data Collection
- Open the Sensor Logger app.
- Select the orientation sensor to log data for roll, pitch, and yaw.
- Start logging and carefully tilt the phone in various directions to capture orientation changes. Continue recording for about 1-2 minutes.
- Save the data and export it as a CSV file from the app.
- Transfer the CSV file from your phone to your computer.
- Open Python and load the data using pandas. Here is sample code to get started:
import matplotlib.pyplot as plt
# Load the CSV file
file = 'Orientation.csv'
try:
data = pd.read_csv(file)
except:
# data file not available, load online file
url = 'http://apmonitor.com/dde/uploads/Main/'
data = pd.read_csv(url+file)
# Cleanse data
data['time'] = (data['time'] - data['time'].iloc[0])/1e9
# Plot roll, pitch, and yaw
plt.figure(figsize=(6,4))
plt.subplot(3,1,1)
plt.plot(data['time'], data['roll'], color='red', label='Roll')
plt.legend()
plt.subplot(3,1,2)
plt.plot(data['time'], data['pitch'], color='blue', label='Pitch')
plt.legend()
plt.subplot(3,1,3)
plt.plot(data['time'], data['yaw'], color='green', label='Yaw')
plt.legend()
plt.xlabel('Time (sec)')
plt.tight_layout()
plt.show()
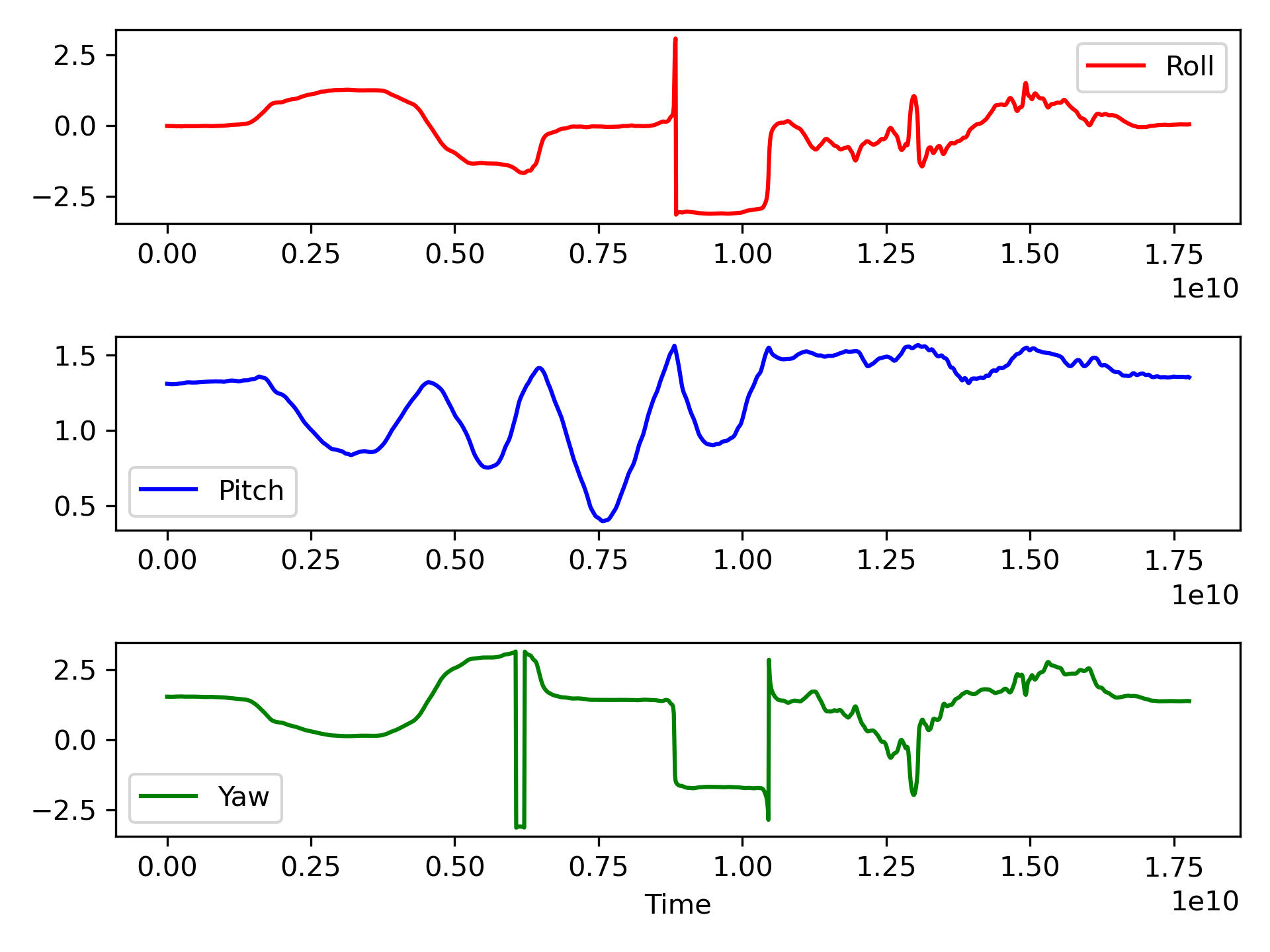
Analysis
Display roll, pitch, and yaw data over time, visualizing the smartphone orientation. Observe any patterns observed in the data. For instance, identify how roll, pitch, and yaw change as the phone orientation varies. This activity teaches data handling and plotting in Python and provides insight into sensor data collection.
✅ Activity: Vibration
Consider how vibration data (acceleration) may be applied in applications like rotating equipment monitoring. Place the phone on a flat surface and start recording. Alternate 5 second periods of tapping the table to induce vibration over about 20 seconds. Plot and analyze the data to show how an accelerometer could be applied to detect excessive vibration from rotating equipment such as a motor or pump.
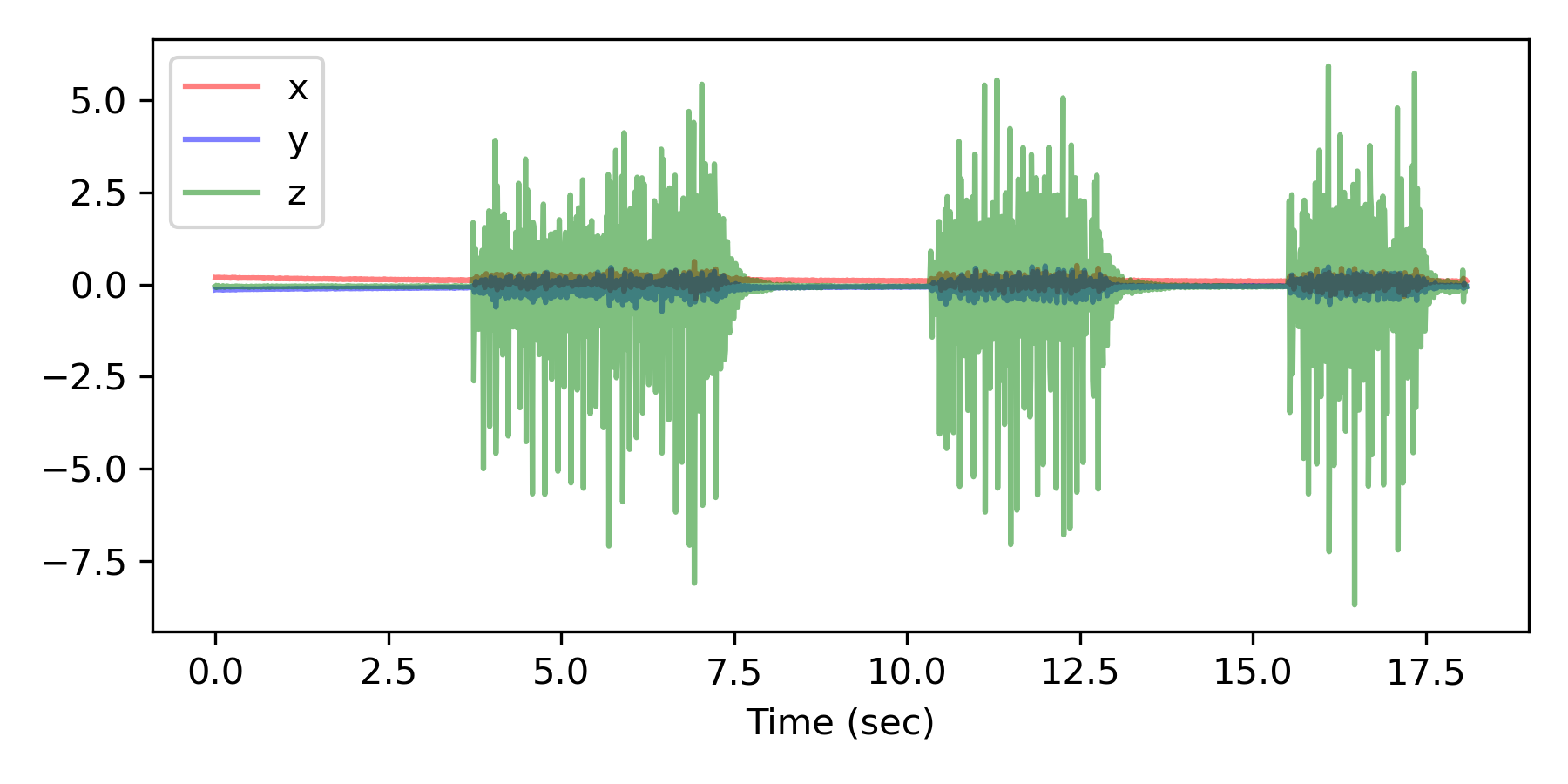
import matplotlib.pyplot as plt
# Load the CSV file
file = 'Accelerometer.csv'
try:
data = pd.read_csv(file)
except:
# data file not available, load online file
url = 'http://apmonitor.com/dde/uploads/Main/'
data = pd.read_csv(url+file)
# Cleanse data
data['time'] = (data['time'] - data['time'].iloc[0])/1e9
# Plot x,y,z acceleration
plt.figure(figsize=(6,3))
plt.plot(data['time'], data['x'], color='red', label='x', alpha=0.5)
plt.plot(data['time'], data['y'], color='blue', label='y', alpha=0.5)
plt.plot(data['time'], data['z'], color='green', label='z', alpha=0.5)
plt.legend()
plt.xlabel('Time (sec)')
plt.tight_layout()
plt.show()
✅ Activity: Cabin Pressure During Flight
Objective: Record and analyze the pressure inside an airplane cabin from takeoff to landing. This activity uses the smartphone barometer sensor to monitor pressure changes during the ascent, cruise, and descent of a flight. The sample demonstration data is for a commercial flight between Atlanta, GA and Tri-Cities, TN.
The difference in elevation is observable as a change in pressure between the two airports and can be used to check the beginning and ending pressures. Atmospheric conditions cause natural variation in the pressure at the same altitude. See the relationships between air pressure and elevation with the BMP280 sensor material.
Data Collection
- Select the barometer in the Sensor Logger app to measure pressure.
- Begin logging data at 10 sec intervals before the aircraft door is closed and continue during the flight. Stop after landing and the aircraft door is opened.
- Save the data as a CSV file and transfer it from the smartphone to a computer.
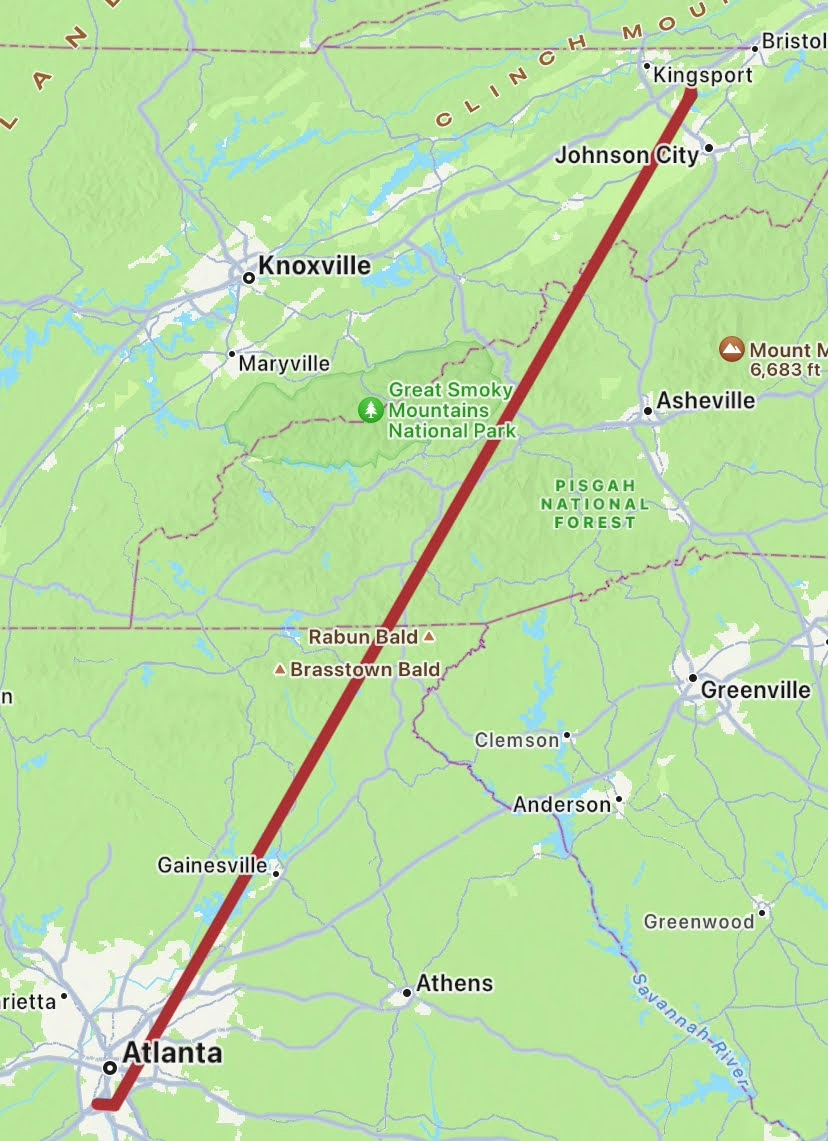
Additional Information on Elevation
- Hartsfield-Jackson Atlanta International Airport is at an elevation of 1,026 feet above sea level.
- Tri-Cities, TN Airport is located at an elevation of 1,519 feet above sea level.
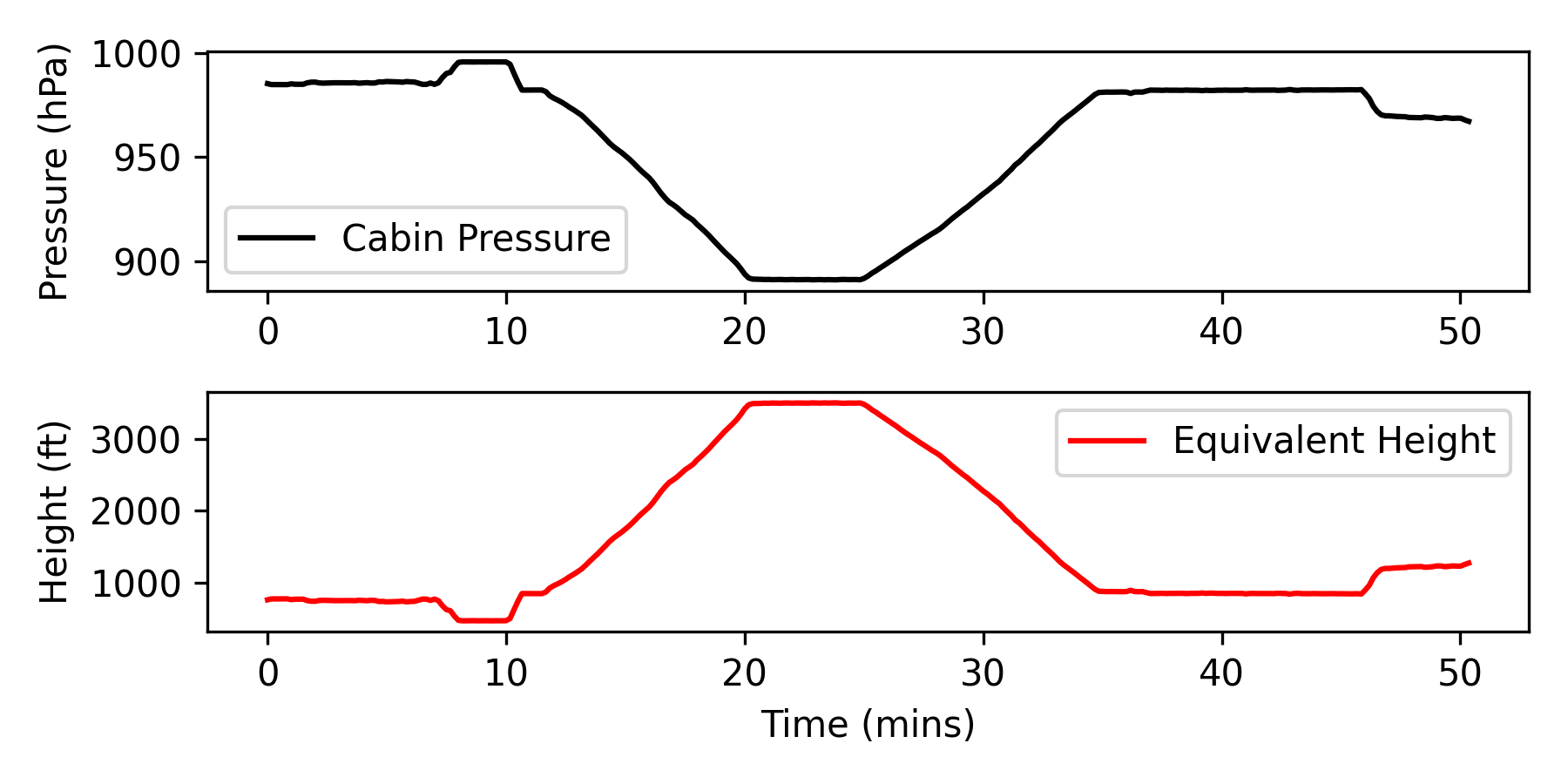
import matplotlib.pyplot as plt
# Load the CSV file
file = 'Barometer.csv'
try:
data = pd.read_csv(file)
except:
# data file not available, load online file
url = 'http://apmonitor.com/dde/uploads/Main/'
data = pd.read_csv(url+file)
# Cleanse data
data['time'] = (data['time'] - data['time'].iloc[0])/60e9
# Equivalent height
T0 = 288.16; L=0.00976; P0=1013.25; Rg=8.31446
g = 9.80665; M=0.02896968
data['h'] = (T0/L)*(1-(data['pressure']/P0)**(Rg*L/(g*M))) * 3.28084
# Plot cabin pressure and equivalent height over time
plt.figure(figsize=(6,3))
plt.subplot(2,1,1)
plt.plot(data['time'], data['pressure'], 'k-', label='Cabin Pressure')
plt.ylabel('Pressure (hPa)'); plt.legend()
plt.subplot(2,1,2)
plt.plot(data['time'], data['h'], 'r-', label='Equivalent Height')
plt.ylabel('Height (ft)'); plt.legend()
plt.xlabel('Time (mins)')
plt.tight_layout()
plt.show()
Additional Information on Elevation
- Hartsfield-Jackson Atlanta International Airport is at an elevation of 1,026 feet above sea level.
- Salt Lake City International Airport is located at an elevation of 4,226 feet above sea level.
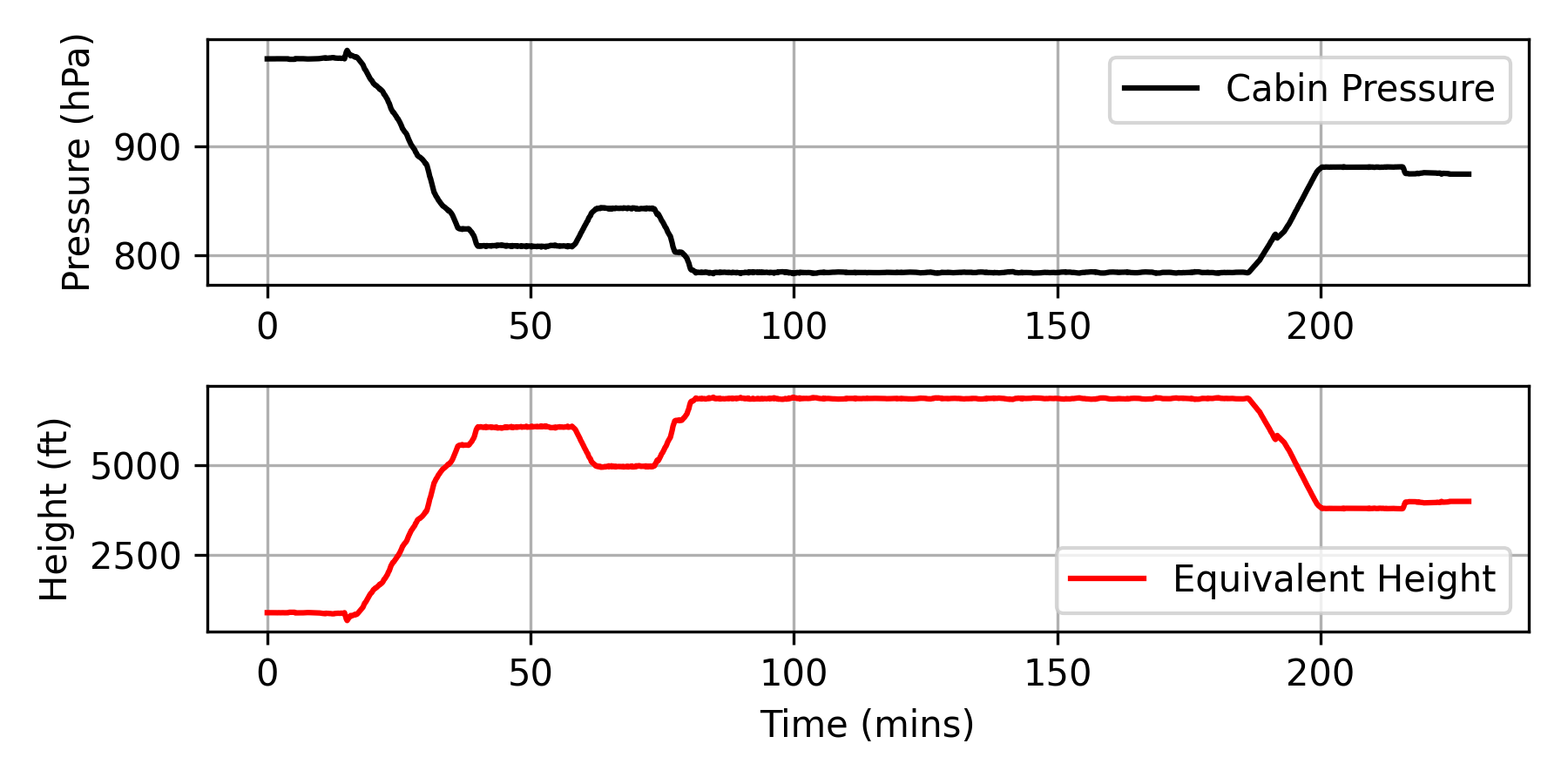
import matplotlib.pyplot as plt
# Load the CSV file
file = 'Barometer2.csv'
url = 'http://apmonitor.com/dde/uploads/Main/'
data = pd.read_csv(url+file)
# Cleanse data
data['time'] = (data['time'] - data['time'].iloc[0])/60e9
# Equivalent height
T0 = 288.16; L=0.00976; P0=1013.25; Rg=8.31446
g = 9.80665; M=0.02896968
data['h'] = (T0/L)*(1-(data['pressure']/P0)**(Rg*L/(g*M))) * 3.28084
# Plot cabin pressure and equivalent height over time
plt.figure(figsize=(6,3))
plt.subplot(2,1,1)
plt.plot(data['time'], data['pressure'], 'k-', label='Cabin Pressure')
plt.ylabel('Pressure (hPa)'); plt.grid(); plt.legend()
plt.subplot(2,1,2)
plt.plot(data['time'], data['h'], 'r-', label='Equivalent Height')
plt.ylabel('Height (ft)'); plt.legend()
plt.xlabel('Time (mins)'); plt.grid()
plt.tight_layout()
plt.show()