👩💻 📚 Python for Data-Driven Engineering
Data-Driven Engineering starts with a foundation of basic tools to import, cleanse, modify, and export data. These seven modules start with an introduction to Python and cover the fundamentals of the language with variable types, tuples, lists, sets, and dictionaries. It explores the commonly used packages Numpy and Pandas. This introductory series gives an understanding of the basics of Python and how to solve data-driven engineering problems.
What is Python?
Python is a powerful, high-level, object-oriented programming language. It was created in 1991 by Guido van Rossum and is used for a wide range of applications. Python is used by many professional software developers, as well as by hobbyists and students. It is a great language for beginners, as it is relatively easy to learn, yet it can be used for complex tasks. Python is a versatile programming language with relatively easy syntax.
Why Python for Data-Driven Engineering?
Python is a great choice for engineers because it can be used for a wide range of data-driven engineering tasks. It can be used for data analysis, numerical computation, visualization, machine learning, automation, and many other applications. Python is also open-source and free, making it an ideal choice for engineers who need to work on a budget. A first step is to run Python either on a local computer or remotely with cloud-computing.
The exercises are available either as a Jupyter Notebook (.ipynb file) on GitHub or through a link to Google Colab.
1️⃣ Python Basics
Data-driven engineering relies on information, often stored in the form of characters (strings) and numbers (integers and floating point numbers). It is essential to import, export, and get data into the correct form so that information can be extracted. This series includes an introduction to Python Basics as foundational elements.
2️⃣ Python Tuple
Tuple (e.g. (i,x,e)) is immutable (does not change) as an efficient storage mechanism for constant sets of values.
3️⃣ Python List
List (e.g. [i,x,e]) is a mutable set of values where it is possible to add elements, remove elements, sort.
4️⃣ Python Set
Set (e.g. {i,x,e}) is a data structure that is similar to list but not sorted and has no duplicate values.
Dictionary (e.g. {'i':i,'x':x,'e':e}) is a data structure with a reference value based on key.
6️⃣ NumPy
NumPy expands upon the basic Python functions to create an array. Matrix and vector operations are designed as a foundation for numerical calculations.
7️⃣ Pandas
Pandas reads, cleanses, calculates, rearranges, and exports data. It is a library for working with data with common high-level functions that simplify the processing steps of analytics and informatics.
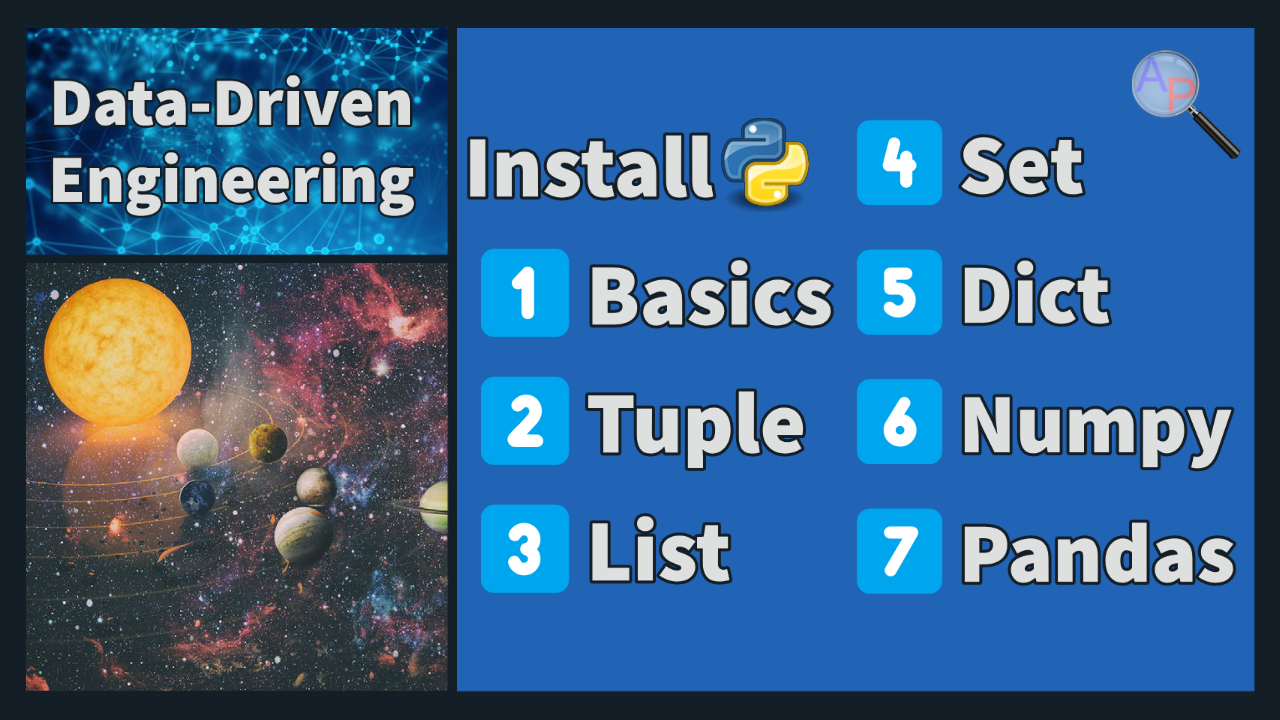