1๏ธโฃ ๐ Python Basics
Python numbers can be stored as a boolean bool (0=False, 1=True), integer int, a floating point number float, or as a string str. A variable name cannot start with a number, has no spaces (use '_' instead), and does not contain a special symbols
' " , < > / ? | \ ( ) ! @ # $ % ^ & * ~ - +
Avoid using reserved Python keywords as variable names:
and assert break class continue def del elif else except exec finally for from global if import in is lambda not or pass print raise return try while
Use the type() function to determine the object type. Run each cell in a Jupyter Notebook with shortcut Shift-Enter.
type(b)
bool
type(i)
int
Multiple numbers can be assigned with a single line.
print(type(x),type(e))
<class 'float'> <class 'float'>
Numbers can also be stored as a string. A string can be created with single 'string' or double quotes "string". Enclose a string in double quotes if a single quote is in the string such as "I'm learning Python".
type(s)
str
โ Operators
- + - * / addition, subtraction, multiplication, division
- % modulo (remainder after division)
- // floor division (discard the fraction, no rounding)
- ** exponential
2.5 1 2 25
๐งญ Comparison Operators
- > greater than, >= or equal to
- < less than, <= or equal to
- == equal to (notice the double equal sign, single assigns a value)
- != or <> not equal to
True False False True
Chained comparisons 5>3>1 can be used as an alternative to 5>3 and 3>1. Use the \ character to continue onto the next line.
5>3 and 3>1)
True True
โ Conditional Statement
Use an if statement to choose code flow based on a True or False result. Indentation (whitespace) is important in Python to indicate which lines belong to the if, elif (else if), or else conditions.
print('x>3')
elif x==3:
print('x==3')
else:
print('x<3')
print(x)
x<3 2.7
โฐ For Loop
Two loop options are for and while. A for loop is used when the number of iterations is known. Use the range(n) function to iterate n times through a for loop.
print(i)
0 1 2
The range function can be used with 1-3 inputs:
- range(n) - iterate n times
- range(start,stop) - iterate between start and stop-1
- range(start,stop,step) - iterate between start and stop-1 with increment step
print(i)
1 3 5
โฟ While Loop
A while loop continues until the condition is False. A while loop is used when the number of cycles is not known until the looping starts. The break and continue statements control the flow in a while loop.
while i<=5:
print(i)
i+=2
1 3 5
Simulate rolling a 6-sided die ๐ฒ by selecting a random number between 1 and 6. Continue until a 3 is rolled. The import random statement makes the randint() function available to generate the random integer number between 1 and 6.
i = None
while i!=3:
i = random.randint(1,6)
print(i)
1 2 5 1 5 4 6 2 2 1 3
๐ Print Numbers
Display a number with the print() function.
2.7
Formatted output controls the way the number is displayed. Display Number: 8.10 with f'Number: {3*x:8.2f}'. The format 8.2f means that there are 8 spaces total and 2 decimal places. The 3*x takes x=2.7 from the cell above and multiplies it by 3.
'Number: 8.10'
Other common format specifiers include:
- d: signed integer
- e or E: floating point exponential format (e=lowercase, E=uppercase)
- f or F: floating point decimal format
- g or G: same as e/E if exponent is >=6 or <=-4, f otherwise
- s: string
The first number 8 indicates how many total spaces in the final string that represents the number. The .2 indicates how many decimal places are displayed. Leave off the 8 to include two decimals with the minimum number of spaces to represent the number (no blanks) with {3*e:.2f}. Strings with extra spaces can be left aligned < or right aligned >. The formatted output of x with 10 spaces is f'{x:>10}'.
' 2.7'
Display 4 decimal places in exponential form with f'{x:.4e}'.
'2.7000e+00'
๐ก Convert Between Types
Use functions to convert between types:
- int() - convert to an integer (no range limits, no decimal)
- float() - convert to a floating point number (no range limits, includes decimal)
- str() - convert to a string (characters, not stored as a number)
- bin() or hex() - convert to binary or hexadecimal form (string)
Python automatically switches from 64-bit to long integer when an integer is greater than 9223372036854775807 (2**63 - 1). Python variables are mutable. Mutable means that the type can change and does not need to be declared before first use. Converting from a float to an int truncates the decimal from 2.7 (float) to 2 (int).
2
Convert x=2.7 to int before converting to binary (base-2) with bin().
'0b10'
Convert x=2.7 to int before converting to hexadecimal (base-16) with hex().
'0x2'
Use round() to get the nearest integer 3 instead of truncating to 2.
3
Adding two string numbers concatenates the two as '1' + '4.9' = '14.9'
'34.9'
Convert the string to a float before adding to get 1 + 4.9 = 5.9. Use s=float(s) to change the class type of s to a float in later cells as well. To change the class type back to a string, use s=str(s). In this case, the i+float(s) changes s to a float only during the operation.
5.9
๐ฉน Try and Except
The error engine stops the code and reports fatal errors. To customize how to handle errors:
# try this code
except Exception:
# with a particular exception
except:
# catch everything else
else:
# do this if there is no exception
finally:
# always run this section at the end
The try and except is an easy way to prevent code from stopping but can mask errors, especially during early development phases when errors are needed to find sytax or logical problems with the code.
x=2/0
except ZeroDivisionError as e:
print(e)
x = None
print('x: ',x)
division by zero x: None
๐ Input Function
A program can be interactive with the input() function to request a value from the user. The value is returned as a string and should be converted to a number with float() or int(), depending on the anticipated entry. Try using the input() function.
๐งถ String Methods
Built-in string methods include:
- upper - convert to uppercase
- lower - convert to lowercase
- find - find index position of first occurance of character or string
- replace - replace string
- split - split sentence into a list of words
Use dir(n) to find other string methods. Replace 'Your Name' with your name.
!replace 'Your Name' with your name
n = n.replace('Name','Your Name')
print(n.lower(), n.upper())
your name YOUR NAME
โฑ Time
Use the time package to get the number of seconds since 1 January 1970. This is a standard reference time that many time packages use.
time.time()
1656198771.4637268
โณ The difference in seconds end-start tracks elapsed clock time.
๐ด The sleep function pauses for a certain amount of time with time.sleep(1) for 1 second. The elapsed time is more than 1.0000 because the time.time() function takes a small amount of time to run.
time.sleep(1)
end = time.time()
print('Elapsed time: ', end-start)
Elapsed time: 1.005829095840454
๐ Date
The datetime is another module to process dates and times. Change the date to a personally memorable date such as a birthdate.
๐ Convert the string to a datetime object with either strptime or fromisoformat if the date is in the standard format YYYY-MM-DD HH:MM:SS.
dt = datetime.fromisoformat(date)
dt
datetime.datetime(1997, 5, 11, 11, 5, 23)
โ Use the now() function to get the current datetime as tm = datetime.now(). Show the current datetime with:
- tm.ctime() - readable format (e.g. Sat Jun 25 17:12:52 2022)
- str(tm) - standard format (e.g. 2022-06-25 17:12:52.536004)
print(tm.ctime())
str(tm)
Sat Jun 25 17:12:52 2022 '2022-06-25 17:12:52.536004'
๐ Elements of the datetime are unpacked with:
- tm.year - year (4 digits)
- tm.month - month (1-12)
- tm.day - day (1-31)
- tm.hour - hour (0-23)
- tm.minute - minute (0-59)
- tm.second - second (0-59)
๐-๐ Calculate elapsed time as a difference diff=tm-dt or convert to elapsed seconds with diff.total_seconds().
print(diff)
print(diff.total_seconds())
9176 days, 6:07:29.536004 792828449.536004
๐ฆ Functions
Two function definitions are:
- lambda - simple one-line functions
- def - code block with input(s) and output(s)
The lambda function is for coding simple one-line expressions. It is especially useful in combination with map(), filter(), and reduce() functions. The lambda function f(x) returns x**2+1. A for loop evaluates the function for values [0,1,2,3].
for i in range(3):
print(i,f(i))
0 1 1 2 2 5
Alternatively, use the def statement to define the f(x) function with the same result.
return x**2+1
for i in range(3):
print(i,f(i))
0 1 1 2 2 5
Multiple inputs and outputs are possible with optional arguments with default (=1) values.
return x*y
print(g(2),g(2,5))
2 10
โGet Help
Use the help() function to access a description of the method or object. Jupyter Notebooks display help with Shift-Tab. Use websites such as Stack Overflow to find answers related error messages.
Help on built-in function lower: lower() method of builtins.str instance Return a copy of the string converted to lowercase.
๐ป Exercise 1A
Change the type of s to a floating point number with s = float(s). Verify that s is a floating point number with print(s) and type(s).
print(s,type(s))
s = float(s)
print(s,type(s))
๐ป Exercise 1B
Ask the user to input an integer with the input() function. Check that the input number is an integer. The try section of the code attempts to convert to a floating point number. The if statement checks if the float number is an integer value. If all conditions are successfully met, the integer is printed.
try:
if not float(r).is_integer():
print('Not an integer')
else:
print('Input Integer: ' + r)
except:
print('Could not convert input (', r, ') to a number')
Use the sample code and test with inputs 1.x, 0, 3. Add a check and an error message if the integer value is outside the range (1-10). Use the code above and modify it to produce an error message if not an integer or outside the range 1-10.
try:
if not float(r).is_integer():
print('Not an integer')
else:
if 1<=float(r)<=10:
print('Input Integer: ' + r)
else:
print('Outside Range (1-10)')
except:
print('Could not convert input (', r, ') to a number')
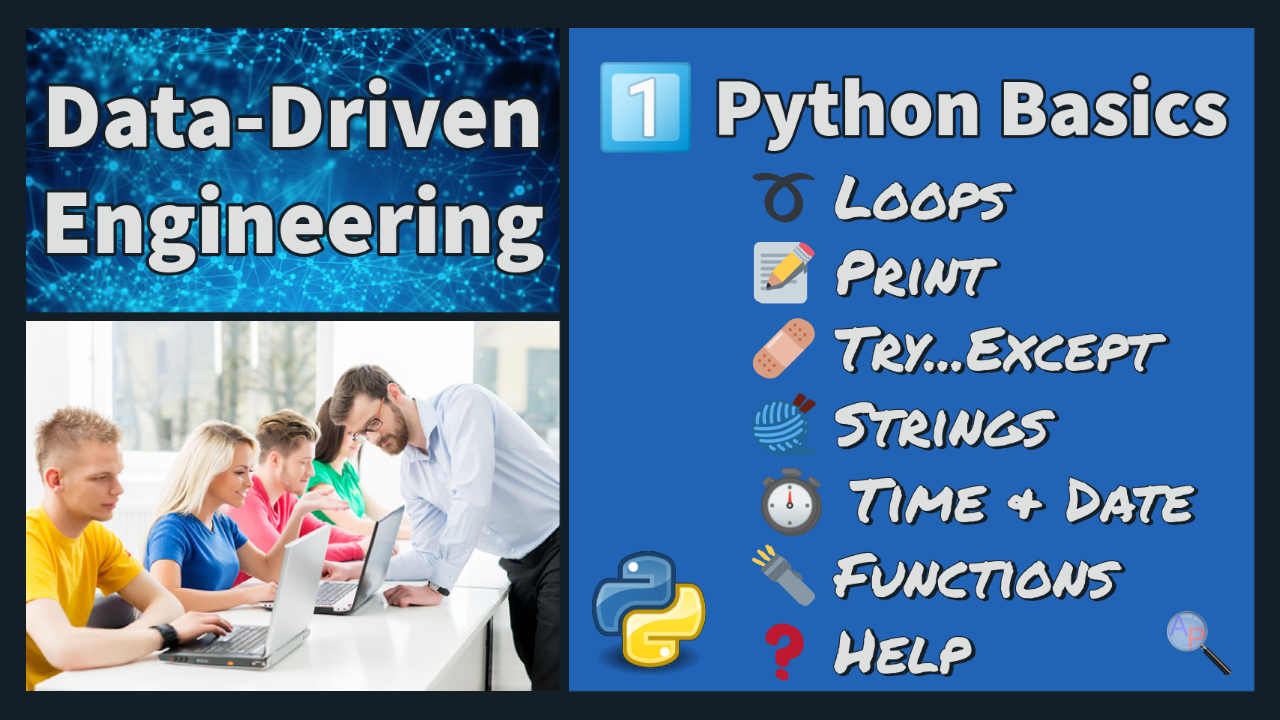
โ Knowledge Check
1. What is the correct way to determine the type of an object in Python?
- Incorrect. In Python, typeof() is not a built-in function to determine the type of an object. Instead, the correct function is type().
- Correct. In Python, the type() function is used to determine the type of an object.
- Incorrect. Python is dynamically typed, which means variable declarations do not specify a type.
- Incorrect. While documentation may provide information about an object, the type() function is the direct way to determine its type.
2. What will the output be for the following code snippet: print(6>2>1) ?
- Incorrect. The code snippet will not produce two boolean values. It will produce a single boolean value as a result of chained comparisons.
- Correct. Chained comparisons in Python are evaluated as individual pairs. In this case, 6>2 and 2>1 are both True, so the entire chained comparison evaluates to True.
- Incorrect. 6>2 and 2>1 are both true comparisons, so the entire chained comparison is True.
- Incorrect. The code snippet is a valid Python syntax using chained comparisons.