4️⃣ 📙 Python Set
A Python set is an unordered collection of unique elements. It is useful because it can perform mathematical set operations such as union, intersection, and difference. Additionally, sets are faster to search than lists or dictionaries because they are implemented using hash tables, which have a constant average-case time complexity for operations such as membership testing and element insertion. Sets are also useful for filtering out duplicates in a list or removing elements from a list that meet certain criteria.
💡 Create Set
Create a set of strings. Unlike a tuple, a set of length 1 is defined without an extra trailing comma {'yes'}. If values are repeated, the duplicate is removed. Empty curly brackets {} create an empty dictionary, not a set. An empty set is defined with t=set().
📝 Print Set
Print the set and verify the object type as a set with the type() function. Because yes appears twice, the duplicate is not added.
type(t)
{'no', 'unknown', 'yes'} set
📑 Unpack Set
Each element of a set is accessed when used as an iterator in a for loop.
print(ti)
no unknown yes
👨👦 Copy Set
Create a copy of set t as u with copy. Using u=t only creates a reference to the original set so changes to u also update t. Use copy to create a new set that is independent of t.
🗑 Remove from Set
Set elements can be removed. Use the remove function to eliminate the element from the list. Use discard to not raise an error if the element is not present. The remove function raises an error if the element is not found.
print(u)
{'no', 'yes'}
🕵️♀️ Inspect Differences
There are methods to investigate differences or create a new set from the differences. Some of these include difference, intersection, issubset, symmetric_difference, and union. The difference function returns the difference with another set.
{'unknown'}
The issubset function returns True if another set contains this set.
True
🔑 Set Attributes and Methods with dir
Use the dir() function to list all attributes (constants, properties) and methods (functions) that are available with an object.
A set has the following methods (functions) with set operators (&,|,-,^,<=,<,>=,>) as a more compact way to compare two sets:
- add - add entry
- clear - clear all entries
- copy - create a copy of the set
- difference (-) - return the difference with another set
- difference_update - remove all elements of another set
- discard - remove an element if it is in the set
- intersection (&) - return the intersection (common elements) of two sets
- intersection_update - update set with the intersection of another
- isdisjoint - return True if two sets have a no common elements
- issubset (<=) - return True if another set contains this set
- issuperset (>=) - return True if this set contains another set
- pop - remove an arbitrary element from set
- remove - remove an element from set
- symmetric_difference (^) - return all elements that are in exactly one of the sets
- symmetric_difference_update - update a set with the symmetric difference between itself and another
- union (|) - return all elements that are in either set
- update - update a set with union of itself and others
A few examples with sets x={-1,0,1,2} and y={1,2,3} demonstrate set operators.
print('x: ',x)
print('y: ',y)
print('Intersection: ', x&y)
print('Difference (x-y): ', x-y)
print('Difference (y-x): ', y-x)
print('Union: ',x|y)
print('Subset: ',x<=y)
print('Superset {0,1,2}>={1,2}:',{0,1,2}>={1,2})
print('Proper Subset: ',x<y)
print('Proper Superset: ',x>y)
x: {0, 1, 2, -1} y: {1, 2, 3} Intersection: {1, 2} Difference (x-y): {0, -1} Difference (y-x): {3} Union: {0, 1, 2, 3, -1} Subset: False Superset {0,1,2}>={1,2}: True Proper Subset: False Proper Superset: False
Because a set is not ordered, there is no particular index for each element. There are also no sort functions. Convert to a list to sort the set.
💻 Exercise 4A
Add entry 'none' to set t. Print the new set.
t.add('none')
print(t)
💻 Exercise 4B
Complete the following steps with set operations.
- Start with a set z={0.5,1,2,4}.
- Create a copy w of set z.
- Remove (pop) an element from w.
- Display the removed element.
print('Original: ',z)
w = z.copy()
w.pop()
print('Modified: ',w)
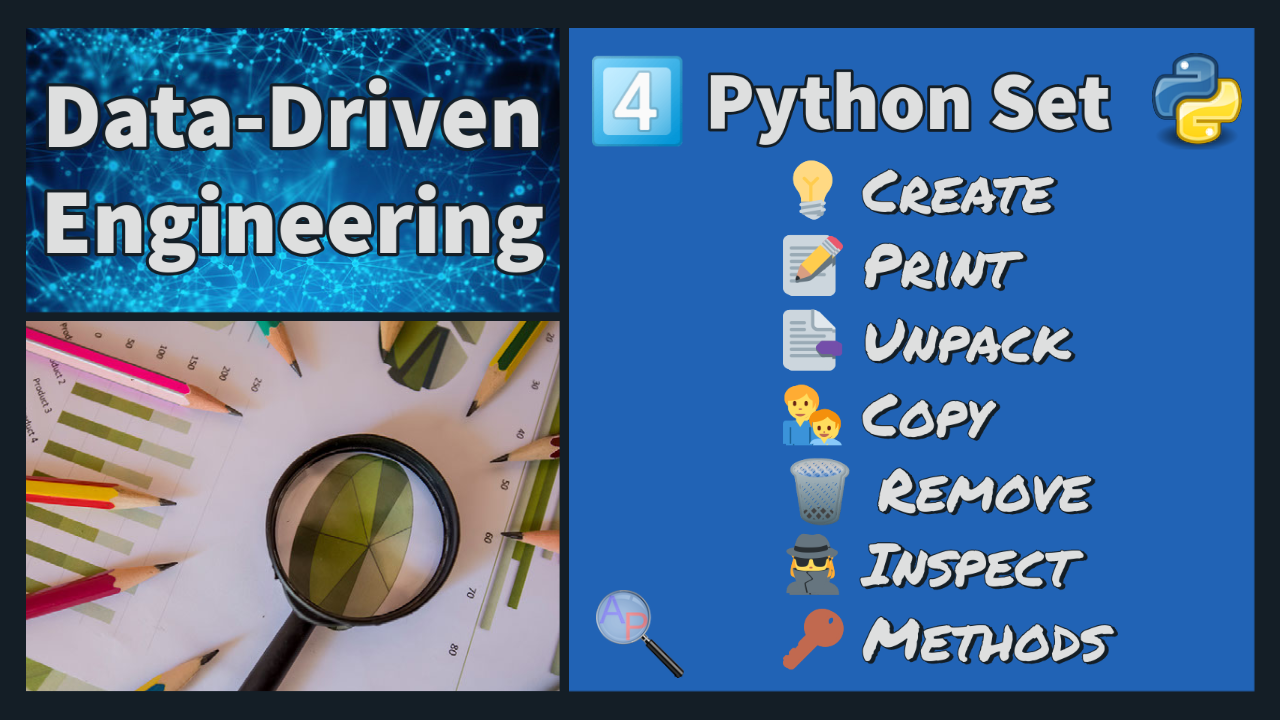
✅ Knowledge Check
1. Which of the following creates an empty set in Python?
- Incorrect. Empty curly brackets {} create an empty dictionary, not a set. An empty set is defined with t=set().
- Correct. This is the correct way to create an empty set in Python.
- Incorrect. This creates a set with a single empty string element, not an empty set.
- Incorrect. This creates an empty list, not an empty set.
2. After executing the code u = t.copy() where t is a set, which statement is true?
- Incorrect. Using the copy method creates a new set that is independent of t. Changes to u will not update t.
- Incorrect. Using the copy method creates a new set that is independent of t. Changes to t will not update u.
- Incorrect. Using the copy method creates a new set that is independent of t. They do not refer to the same memory location.
- Correct. This is correct. Using the copy method creates a new set that is independent of t. Both sets have the same elements, but they are distinct sets.