Optimization Modeling Languages
Two tools used in this course are MATLAB and Python. Python Gekko is a package within Python to provide support for solution of dynamic simulation, estimation, and control.
There are many tools for simulation and optimization of dynamic systems. There are also many factors to consider when selecting the appropriate tool for a particular task. Some of the considerations for a dynamic optimization tool are size (scaling with number equations and degrees of freedom), speed (use in real-time estimation or control), usability (training requirements and ease of adaptation), extensibility (ability to modify for new or complex systems), support (commercial sustainability or open-source community), and portability (ability to embed on a system or communicate with a particular platform).
Here is a list of some of the most popular optimization packages in Matlab:
- fmincon: This is a built-in function in Matlab that implements a variety of optimization algorithms for constrained nonlinear optimization.
- fminunc: This is another built-in function in Matlab that implements a variety of optimization algorithms for unconstrained nonlinear optimization.
- linprog: This is a built-in function in Matlab that implements linear programming algorithms for solving optimization problems with linear constraints.
- quadprog: This is a built-in function in Matlab that implements quadratic programming algorithms for solving optimization problems with quadratic objective functions and linear constraints.
- Global Optimization Toolbox: This is a toolbox in Matlab that provides a variety of algorithms for global optimization, including genetic algorithms, particle swarm optimization, and simulated annealing.
Here is a list of some of the most popular optimization packages in Python:
- scipy.optimize: This is a module in the SciPy library that provides a variety of optimization algorithms for solving nonlinear optimization problems.
- cvxpy: This is a package that provides a modeling language for convex optimization problems, and it has interfaces to several solvers including CVXOPT, ECOS, and SCS.
- gekko: This is a package that provides a modeling language for linear, nonlinear, and mixed integer programming problems, and it has interfaces to several solvers including IPOPT, APOPT, and BPOPT.
- pygmo: This is a package that provides a variety of optimization algorithms for global optimization, including genetic algorithms, particle swarm optimization, and simulated annealing.
- PyOpt: This is a package that provides a variety of optimization algorithms for solving nonlinear optimization problems, including gradient-based and derivative-free methods.
The objective of this section is to introduce optimization in MATLAB and Python. This is not intended to be a general tutorial but more specifically to cover topics that are relevant for dynamic optimization. Topics of interest for dynamic optimization are listed below.
Introduction to Nonlinear Optimization
This course uses principles from nonlinear programming but does not delve into the details of solution strategies. Instead of detailing how a nonlinear programming solver iterates to find a solution, this course focuses on formulating problems and the use of differential constraints in describing and optimizing dynamic systems. For more background on how nonlinear programming solvers work, refer to the Course on Optimization and the associated online Design Optimization Textbook. A good illustrative example is the Two Bar Truss optimization or the example below.
GEKKO (Python)
GEKKO Python is designed for large-scale optimization and accesses solvers of constrained, unconstrained, continuous, and discrete problems. Problems in linear programming, quadratic programming, integer programming, nonlinear optimization, systems of dynamic nonlinear equations, and multi-objective optimization can be solved. The platform can find optimal solutions, perform tradeoff analyses, balance multiple design alternatives, and incorporate optimization methods into external modeling and analysis software. It is free for academic and commercial use under the MIT license.
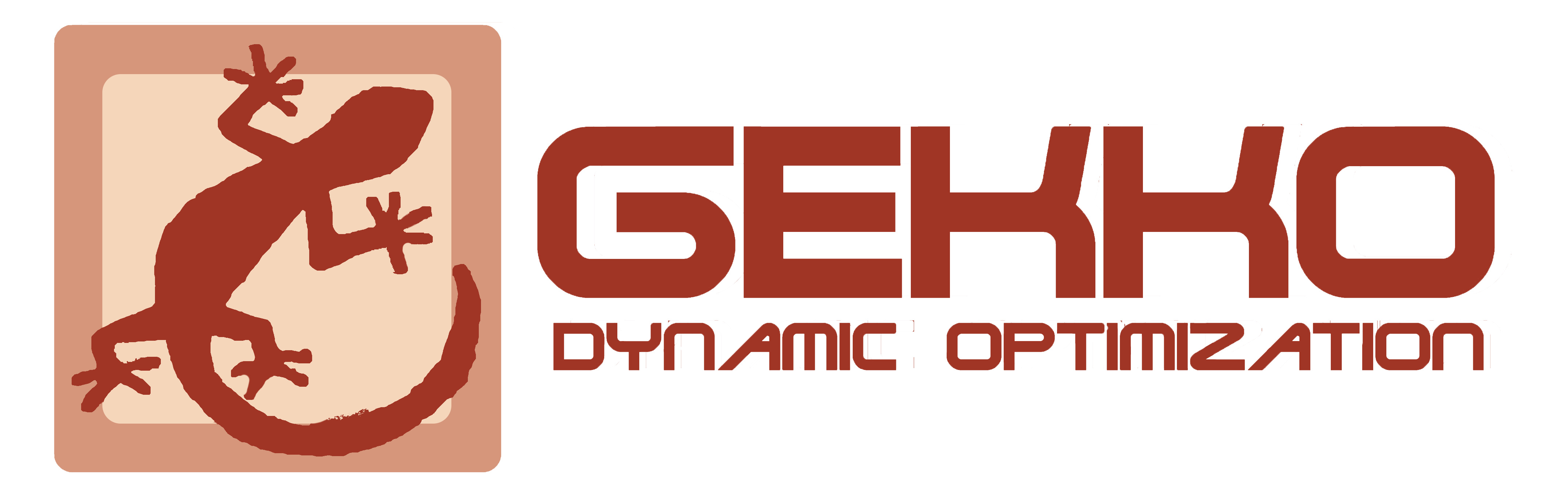
The GEKKO package is available through the package manager pip in Python.
View Source for 18 GEKKO Example Solutions
APMonitor (MATLAB and Python)
APMonitor is a tool for nonlinear optimization and dynamic optimization. Source files are available for Julia, Python, and MATLAB. Users can select either a public server or a local host if the APMonitor Server is installed locally. The user must also specify an application name.
server = 'https://127.0.0.1' # for local server server = 'https://byu.apmonitor.com' # for public server app = myApp # application name
Once the server and application name are defined, the following is a list of available commands in each package.
- apm(server,app,aline) - Send commands to the server
- Typical commands are 'clear all', 'clear csv', 'clear apm', 'solve'.
- 'clear all': Clear the prior application
- 'clear csv': Clear just the data file (CSV)
- 'clear apm': Clear just the model file (APM)
- 'solve': Solve the problem
- apm_get(server,app,filename) - Retrieve a file from the server
- apm_info(server,app,type,aline) - Classify a parameter or variable]]
- More information about FV, MV, SV, and CV types
- Type FV: Fixed value, one decision or fixed value over the time horizon
- Type MV: Manipulated variable, multiple decision values over the horizon
- Type SV: State variable, view in web-viewer but don't control
- Type CV: Controlled variable with target variable
- apm_ip(server) - Retrieve the client IP address
- Example: ip = apm_ip('https://apmonitor.com')
- apm_load(server,app,filename) - Load model file
- apm_meas(server,app,name,value) - Specify a measurement
- apm_option(server,app,name,value) - Set an option
- apm_sol(server,app) - Retrieve the solution
- apm_tag(server,app,name) - Retrieve an NLC, FV, MV, SV, or CV option
- apm_web(server,app) - Open a web interface to the dashboard
- apm_web_var(server,app) - Open a web interface to the variable values
- csv_load(server,app,filename) - Load data file
An example script is below. The same commands are used in Python, MATLAB, and Julia with minor modifications such as the ';' in MATLAB to suppress console output or change in the comment characters.
# https://apmonitor.com/wiki/index.php/Main/PythonApp
from apm import *
# for MATLAB use: addpath('apm')
s = 'https://byu.apmonitor.com'
a = 'myApp'
# clear prior application
apm(s,a,'clear all')
# load model and data
apm_load(s,a,'model.apm')
csv_load(s,a,'data.csv')
# specify FV, MV, SV, CV
apm_info(s,a,'FV','K')
apm_info(s,a,'MV','u')
apm_info(s,a,'SV','x')
apm_info(s,a,'CV','y')
# configuration parameters
apm_option(s,a,'nlc.imode',6)
apm_option(s,a,'nlc.nodes',3)
# turn on MV as a degree of freedom
apm_option(s,a,'u.status',1)
# turn on CV to add terms to objective function
apm_option(s,a,'y.status',1)
# tune MV in the controller
apm_option(s,a,'u.lower',0)
apm_option(s,a,'u.upper',100)
apm_option(s,a,'u.dcost',0.1)
# tune CV in the controller
apm_option(s,a,'y.tau',5)
apm_option(s,a,'y.sphi',26)
apm_option(s,a,'y.splo',24)
# solve and retrieve results
output = apm(s,a,'solve')
print(output)
# open web-viewer
apm_web(s,a)
# retrieve solution
z = apm_sol(s,a)