Orthogonal Collocation on Finite Elements
Discretization of a continuous time representation allow large-scale nonlinear programming (NLP) solvers to find solutions at specified intervals in a time horizon.

There are many names and related techniques for obtaining mathematical relationships between derivatives and non-derivative values. Some of the terms that are relevant to this discussion include orthogonal collocation on finite elements, direct transcription, Gauss pseudospectral method, Gaussian quadrature, Lobatto quadrature, Radau collocation, Legendre polynomials, Chebyshev polynomials, Jacobi polynomials, Laguerre polynomials, any many more. There are many papers that discuss the details of the derivation and theory behind these methods1-5. The purpose of this section is to give a practical introduction to orthogonal collocation on finite elements with Lobatto quadrature for the numerical solution of differential algebraic equations. See the documentation on Nodes for additional details on displaying the internal nodes.
Exercise 1
Objective: Solve a differential equation with orthogonal collocation on finite elements. Create a MATLAB or Python script to simulate and display the results. Estimated Time: 2-3 hours
Solve the following differential equation from time 0 to 1 with orthogonal collocation on finite elements with 4 nodes for discretization in time.
5 dx/dt = -x2 + u
Specify the initial condition for x as 0 and the value of the input, u, as 4. Compare the solution result with 2-6 time points (nodes). Report the solution at the final time for each and comment on how the solution changes with an increase in the number of nodes.
Solution 1
Exercise 2
Compare orthogonal collocation on finite elements with 3 nodes with a numerical integrator (e.g. ODE15s in MATLAB or ODEINT in Python). Calculate the error at each of the solution points for the equation (same as for Exercise 1):
5 dx/dt = -x2 + u
Solution 2
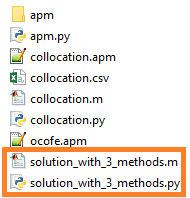
References
- Hedengren, J. D. and Asgharzadeh Shishavan, R., Powell, K.M., and Edgar, T.F., Nonlinear Modeling, Estimation and Predictive Control in APMonitor, Computers & Chemical Engineering, Volume 70, pg. 133–148, 2014. Available at: ScienceDirect or ResearchGate
- Cizniar, M., Salhi, D., Fikar, M., and Latifi, M.A., A Matlab Package for Orthogonal Collocations on Finite Elements in Dynamic Optimization, 15th Int. Conference Process Control, June 7-10, 2015. Article
- Renfro, J., Morshedi, A., Asbjornsen, O., Simultaneous Optimization and Solution of Systems Described by Differential/Algebraic Equations, Computers & Chemical Engineering, Volume 11, Number 5, pp. 503-517, 1987.
- Finlayson, B., Orthogonal Collocation on Finite Elements for PDE Discretization and Solution
- Biegler, L.T., Solution of Dynamic Optimization Problems by Successive Quadratic Programming and Orthogonal Collocation, Computers & Chemical Engineering, Volume 8, Number 3/4, pp. 243-248, 1984.
- Kameswaran, S., Biegler, L.T., Convergence rates for direct transcription of optimal control problems using collocation at Radau points, Computational Optimization and Applications, 41 (1), pp. 81-126, 2008, DOI: 10.1007/s10589-007-9098-9.