Lotka Volterra Fishing Optimization
The Lotka Volterra fishing problem is a Mixed Integer Optimal Control Problem (MIOCP). It finds a fishing strategy over 12 years to equilibrate the predator-prey fish to a sustainable steady-state value. The Lotka Volterra equations for a predator-prey system have an additional term to introduce fishing by man with constants and .
The differential states and are the biomass of prey and predator, respectively. The third differential state is the integral of the squared error to drive both the predator and prey biomass values to 1.0. The decision to send out the fishing fleet at time is the manipulated variable . The time window is from 0 to 12.
The Lotka Volterra fishing problem seeks an optimal fishing strategy over a fixed time horizon to bring both predator and prey fish to a desired steady state. The manipulated variable is the fishing by man. The manipulated variable is either continuous or a discrete value with no fishing (0) or full fishing (1).
The mathematical equations are Ordinary Differential Equations (ODEs). The optimal binary manipulated variable chatters on and off, making the Lotka Volterra fishing problem an interesting benchmark of mixed-integer optimal control solvers.
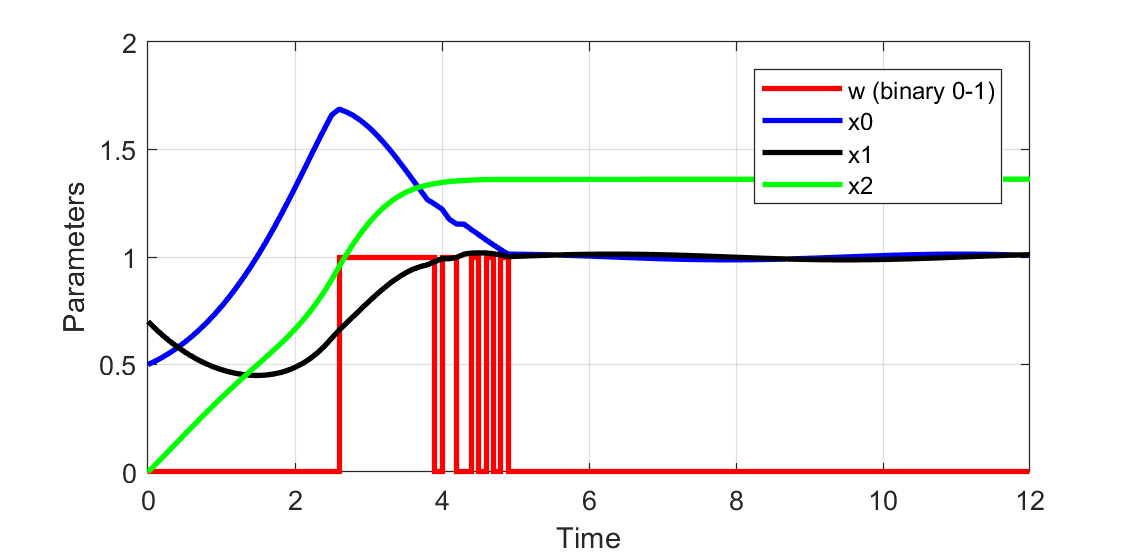
An MINLP solution is calculated with APOPT with an objective function value of .
Avoid Actuator Chatter
The solution with a squared objective has chatter in the fishing decision variable w as the fishing fleet is deployed and returns repeatedly to achieve a perfect balance between predator and prey. This repeated action can wear out an actuator such as a valve that is quickly switched open or closed or a motor that switched on or off. The fishing fleet deployment schedule would also have additional costs for the fishermen and logistics to implement the schedule. A potential improvement is to set up a dead-band around the objective or to include actuator move-suppression. A dead-band of +/-0.05 is set around the objective of 1.0 so that 0.95 to 1.05 are acceptable for the predator and prey levels.
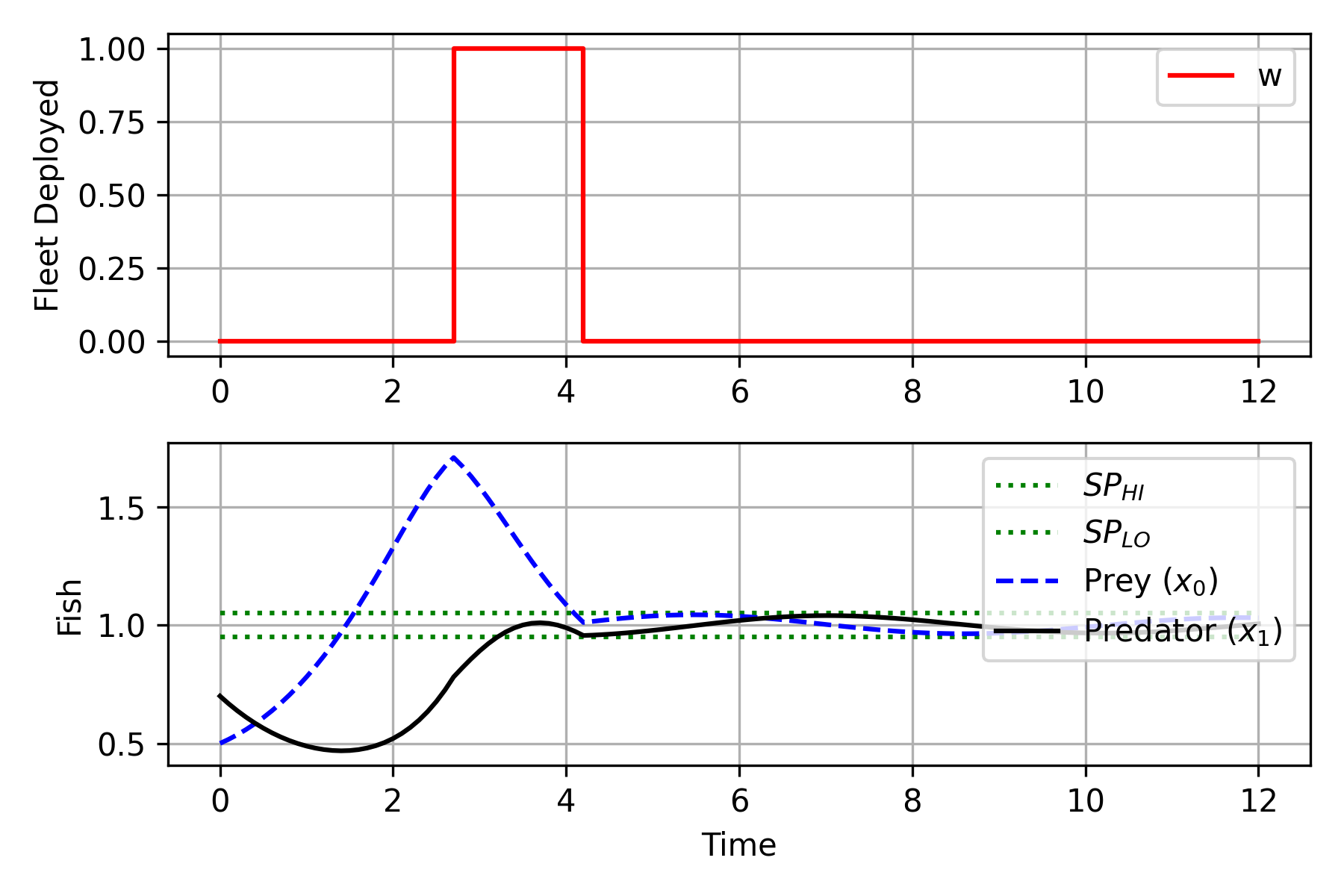
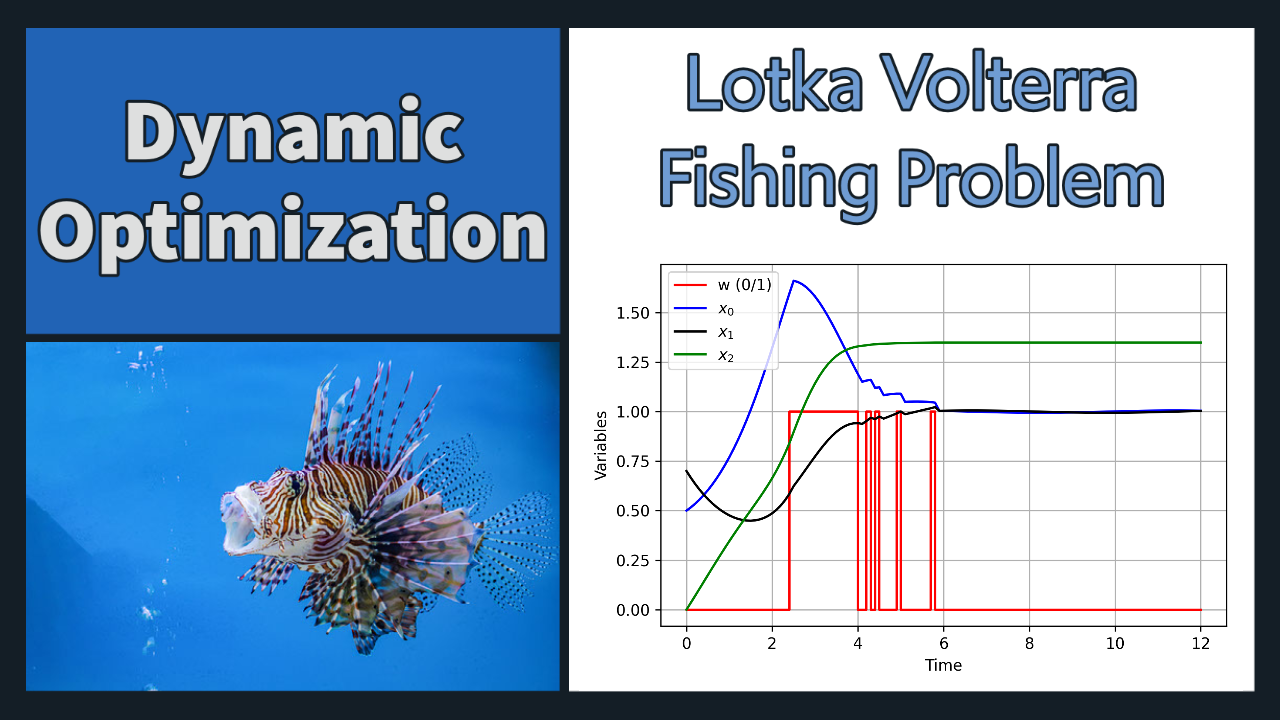
Reference
- Sager, S., Bock, H.G., Diehl, M., Reinelt, G., Schloder, J.P. (2006). Numerical Methods for Optimal Control with Binary Control Functions Applied to a Lotka-Volterra Type Fishing Problem. In: Seeger, A. (eds) Recent Advances in Optimization. Lecture Notes in Economics and Mathematical Systems, vol 563. Springer, Berlin, Heidelberg. https://doi.org/10.1007/3-540-28258-0_17
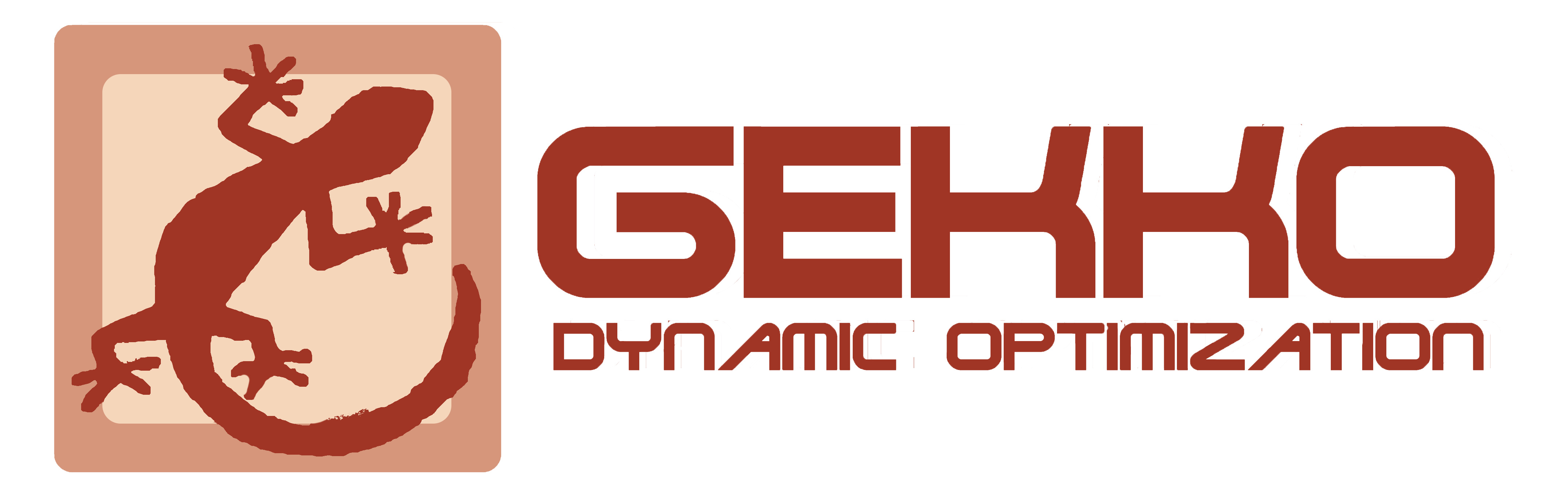
The Gekko Optimization Suite is a machine learning and optimization package in Python for mixed-integer and differential algebraic equations. The GEKKO package is available in Python with pip install gekko. There are additional example problems for equation solving, optimization, regression, dynamic simulation, model predictive control, and machine learning. Cite Gekko Paper