Temperature MAX6675
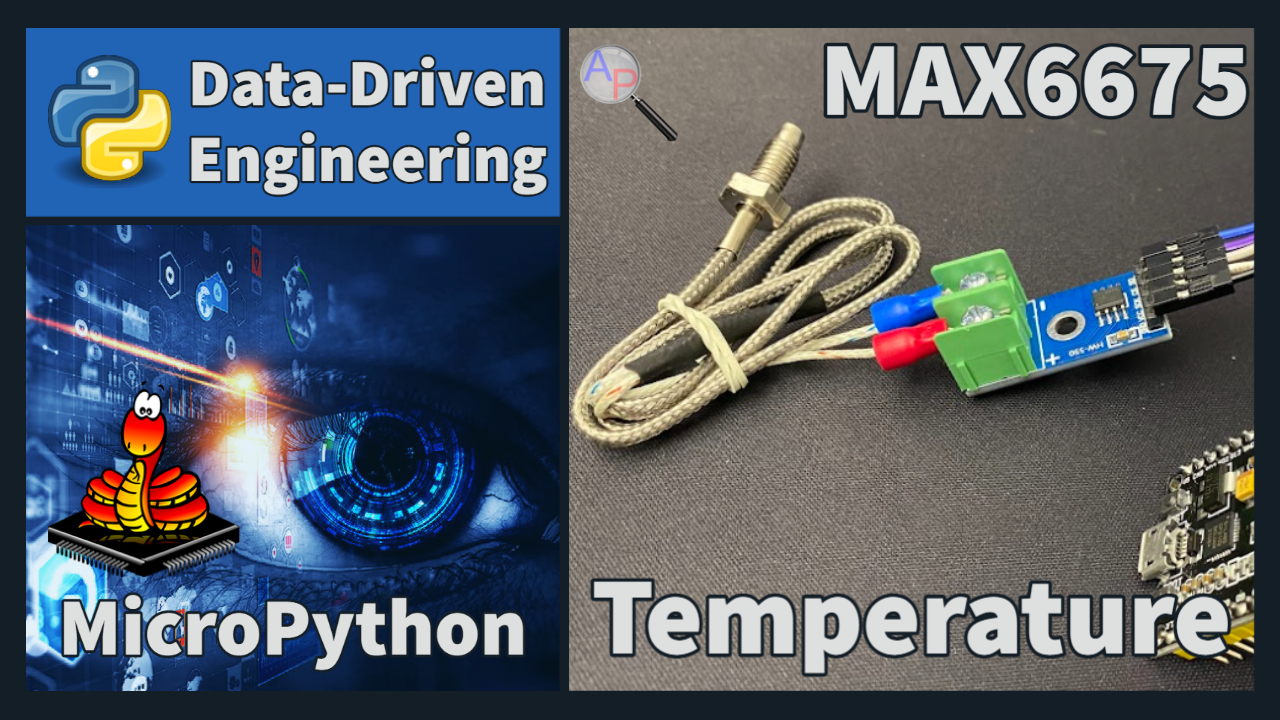
Temperature is an important physical quantity that is widely measured and controlled in a variety of applications. Accurate temperature measurement and control can be critical for ensuring the proper functioning of a system or for maintaining the quality and safety of products. There are many different types of sensors that can be used to measure temperature, including those that use thermocouples, resistance temperature detectors (RTDs), and thermistors. The MAX6675 signal conditioner and thermocouple is a device that is specifically designed to be used with a microcontroller, such as an Arduino or ESP32.
A thermocouple is a type of temperature sensor that consists of two different types of metal wire that are joined together at one end. A voltage is generated that is proportional to the temperature. The voltage generated by the thermocouple is very small, typically on the order of millivolts, and it is amplified using a signal conditioner. In this case, the MAX6675 converts the voltage differential into a digital signal that is read by a microcontroller. Thermocouples are widely used for temperature measurement and control because they are relatively inexpensive and can be used to measure a wide range of temperatures, from very low to very high. They are also relatively rugged and can withstand harsh environments, making them suitable for use in industrial and scientific applications.
One of the main limitations of thermocouples is that they are not very accurate. The voltage generated by a thermocouple can vary depending on the type of metal used and the quality of the thermocouple, and the accuracy of the temperature measurement can also be affected by other factors such as electromagnetic interference. Despite these limitations, thermocouples are still widely used because they are low cost and versatile.
MAX6675 Thermocouple-to-Digital Converter
The MAX6675 is a thermocouple-to-digital converter that is designed to measure temperature using a thermocouple sensor. It is a small, 8-pin integrated circuit (IC) that can be easily connected to a microcontroller or other digital device using standard communication protocols such as SPI (serial peripheral interface).
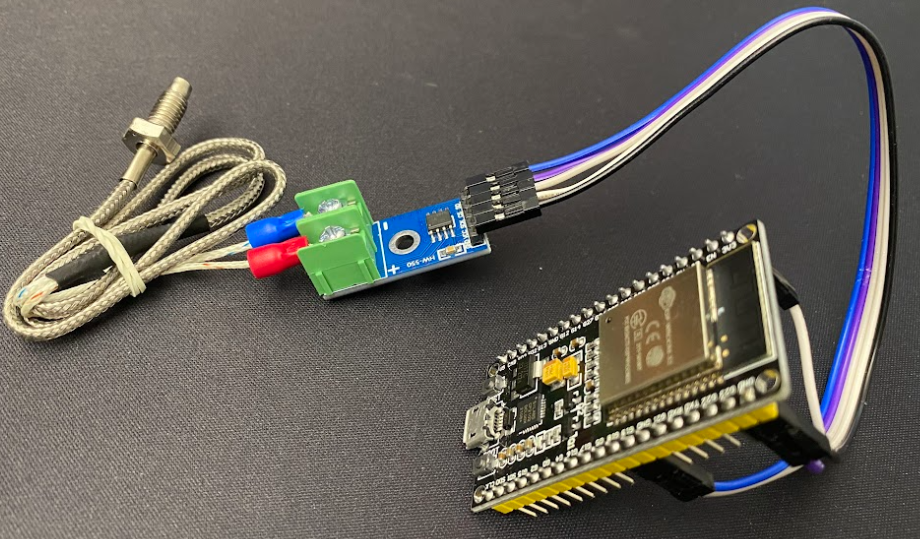
The MAX6675 is designed to work with a wide range of thermocouple types, including K-type, J-type, and T-type thermocouples. It has a temperature measurement range of 0°C to 1024°C and an accuracy of ±2°C. The MAX6675 can be used to measure temperature with a resolution of 0.25°C with a 12-bit number output. It has a built-in cold junction compensation circuit that helps to improve the accuracy of the temperature measurement.
One of the main advantages of the MAX6675 is that it can be easily integrated into a temperature measurement system using a microcontroller or other digital device. It provides a digital output that can be read by the microcontroller. This makes it an attractive option for applications where the temperature measurement needs to be integrated into a digital control system. Micropython code collects pressure data from the MAX6675 sensor.
import time
T = MAX6675(sck_pin=18, cs_pin=23, so_pin=19)
for _ in range(20):
print(T.read())
time.sleep(1)
The max6675.py script is required to access the MAX6675 temperature readings and return the temperature. Thanks to Ivan Sevcik for providing an initial version of the library.
from machine import Pin
class MAX6675:
MEAS_MS = 220
def __init__(self, sck_pin=18, cs_pin=23, so_pin=19):
sck = Pin(sck_pin, Pin.OUT)
cs = Pin(cs_pin, Pin.OUT)
so = Pin(so_pin, Pin.IN)
self._sck = sck
self._sck.value(0)
self._cs = cs
self._cs.value(1)
self._so = so
self._so.value(0)
self._last_meas_start = 0
self._last_read_temp = 0
self._error = 0
def _cycle_sck(self):
self._sck.value(1)
time.sleep_us(1)
self._sck.value(0)
time.sleep_us(1)
def refresh(self):
"""
Start a new measurement.
"""
self._cs.value(0)
time.sleep_us(10)
self._cs.value(1)
self._last_meas_start = time.ticks_ms()
def ready(self):
"""
Signals if measurement is finished.
:return: True if measurement is ready for reading.
"""
elapsed = time.ticks_ms() - self._last_meas_start
return elapsed > MAX6675.MEAS_MS
def error(self):
"""
Returns error bit of last reading.
If this bit is set (=1), there's problem with the
thermocouple - it can be damaged or loosely connected
:return: Error bit value
"""
return self._error
def read(self):
"""
Reads last measurement and starts a new one.
If new measurement is not ready yet, returns last value.
Note: The last measurement can be quite old
To refresh measurement, call refresh and wait for
ready to become True before reading.
:return: Measured temperature
"""
# Check if new reading is available
if self.ready():
# Bring CS pin low to start protocol for reading result of
# the conversion process. Forcing the pin down outputs
# first (dummy) sign bit 15.
self._cs.value(0)
time.sleep_us(10)
# Read temperature bits 14-3 from MAX6675.
value = 0
for i in range(12):
# SCK should resemble clock signal and new SO value
# is presented at falling edge
self._cycle_sck()
value += self._so.value() << (11 - i)
# Read the TC Input pin to check if the input is open
self._cycle_sck()
self._error = self._so.value()
# Read the last two bits to complete protocol
for i in range(2):
self._cycle_sck()
# Finish protocol and start new measurement
self._cs.value(1)
self._last_meas_start = time.ticks_ms()
self._last_read_temp = value * 0.25
return self._last_read_temp

✅ Activity: HVAC Temperature Measurements
Here are a few examples of applications where an temperature sensor might be used:
- Control: An Arduino temperature sensor can be used to control the temperature of a system or process by activating or deactivating heating or cooling elements based on the measured temperature. For example, a temperature sensor could be used to control the temperature of a greenhouse, an incubator, or a bioreactor.
- Alerts: A temperature sensor can be used to monitor the temperature of a system or process and alert the user if the temperature falls outside of a predetermined range. This could be used, for example, to monitor the temperature of a server room or a laboratory and alert the user if the temperature becomes too high or too low.
- Research: A thermocouple can be used as part of a scientific or research project to measure and record temperature data. For example, a temperature sensor could be used to measure the temperature of soil as part of a study on plant growth.
- Industrial: Temperature sensors can be used in industrial settings to monitor and control the temperature of various processes and systems. For example, a temperature sensor could be used to monitor a polymer reactor to prevent run-away reaction.
Use the MAX6675 module with the thermocouple to record temperature measurements of a heating or cooling duct output as it cycles on and off. Explain what you observed about the accuracy and resolution of the measurements. What did the temperature measurements reveal about the HVAC system as it cycled on and off?