MQTT Communication
MQTT (Message Queue Telemetry Transport) is a protocol for machine-to-machine communication that is popular in IoT (Internet of Things) networks. The broker manages data transport from clients that publish or subscribe to updates. It was created around 1998 and has increased in popularity with the increase of IoT devices. It is especially good for ad hoc networking of devices that have varying levels of latency and communication bandwidth. MQTT typically runs over TCP/IP for bi-directional and lossless connections.
MQTT Broker (Server)
The MQTT broker can be in the cloud or hosted locally. The amqtt library is a lightweight Python option for hosting a broker locally.
Create the MQTT broker with the following Python script. This script runs indefinitely to manage the publish/subscribe requests from clients.
Run MQTT Broker
import os
from amqtt.broker import Broker
config = {
"listeners": {
"default": {
"type": "tcp",
"bind": "0.0.0.0:1883",
},
"ws-mqtt": {
"bind": "127.0.0.1:8080",
"type": "ws",
"max_connections": 10,
},
},
"sys_interval": 10,
"auth": {
"allow-anonymous": True
},
"topic-check": {"enabled": False},
}
broker = Broker(config)
async def test_coro():
await broker.start()
if __name__ == "__main__":
asyncio.get_event_loop().run_until_complete(test_coro())
asyncio.get_event_loop().run_forever()
Another broker option is to download Eclipse Mosquitto or use the online MQTT broker.
MQTT Client with Local Broker
The paho-mqtt package is a popular Python MQTT client. Paho also provides MQTT client libraries for other languages as well. Install the Paho client with pip. Also install the tclab library to provide an Internet of Things (IoT) device or emulator.
pip install tclab
This example uses the amqtt local broker with the paho client. Use tclab.TCLab() if a TCLab physical device is connected. Otherwise, use the TCLab digital twin with tclab.TCLabModel().
Client to Publish Temperature to Local Broker
import time
import tclab
mqttBroker ='127.0.0.1'
client = mqtt.Client('TCLab')
client.connect(mqttBroker,port=8080)
with tclab.TCLabModel() as lab:
lab.Q1(70) # set heater 1 to 70%
# cycle 30 sec, publishing temperature
for i in range(30):
client.publish('T1', lab.T1)
print('Published ' + str(round(lab.T1,2)))
time.sleep(1)
MQTT Client with Cloud Broker
This example uses the online MQTT broker provided by Eclipse Mosquitto from the Eclipse Foundation. It is an open-source message broker that can also be installed locally. There are also premium services such as Cedalo and Streamsheets for dashboarding. Test simple MQTT clients that publish and subscribe to the Eclipse Mosquitto public MQTT broker.
Client to Publish Temperature to Cloud Broker
import time
import tclab
mqttBroker ='mqtt.eclipseprojects.io'
client = mqtt.Client('TCLab')
client.connect(mqttBroker)
# use tclab.TCLab() if physical device connected
# otherwise use digital twin tclab.TCLabModel()
with tclab.TCLabModel() as lab:
lab.Q1(70) # set heater 1 to 70%
# cycle 30 sec, publishing temperature
for i in range(30):
client.publish('T1', lab.T1)
print('Published ' + str(round(lab.T1,2)))
time.sleep(1)
Client to Subscribe to Temperature through Cloud Broker
import struct
def on_message(client, userdata, msg):
print(f'Temperature: {float(msg.payload)}')
mqttBroker ='mqtt.eclipseprojects.io'
client = mqtt.Client('IoT_Display')
client.on_message = on_message
client.connect(mqttBroker)
client.subscribe('T1')
client.loop_forever()
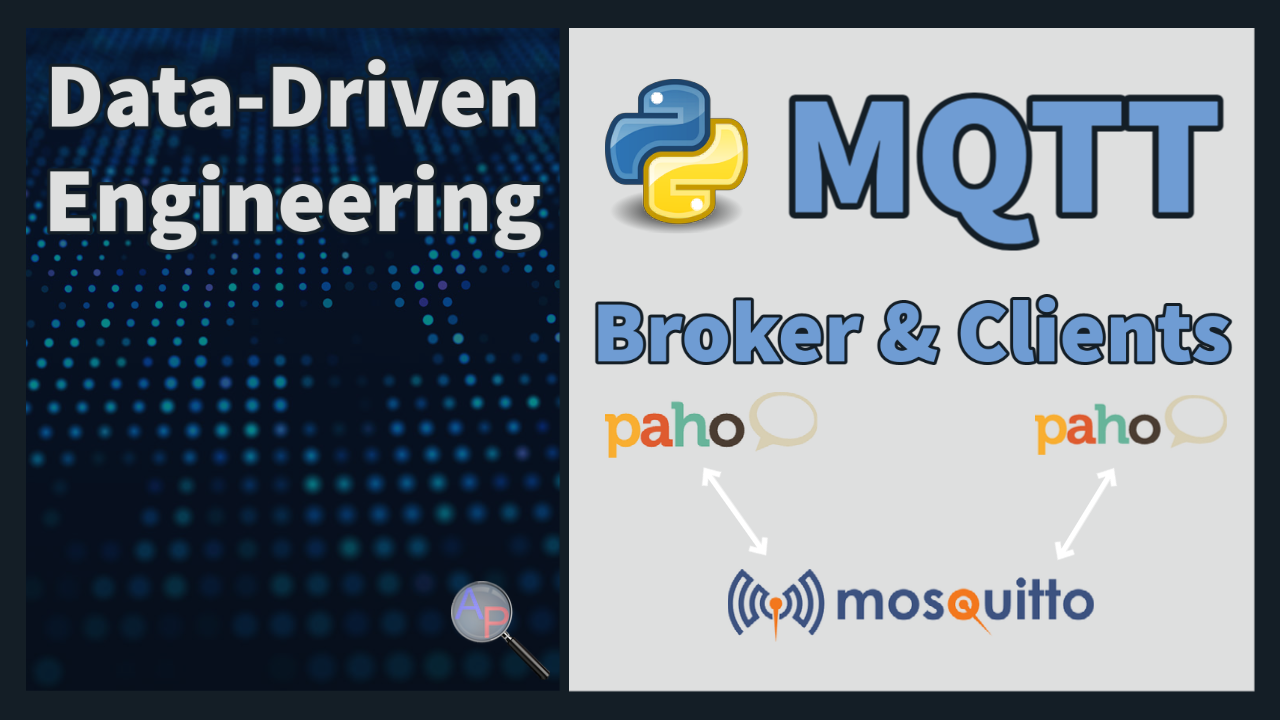
✅ Knowledge Check
1: What does MQTT stand for?
2: Which library can be used to host an MQTT broker locally in Python?