Optimization with Python
Main.PythonOptimization History
Show minor edits - Show changes to markup
<iframe width="560" height="315" src="//www.youtube.com/embed/Cn91MrBbxqc?list=PLLBUgWXdTBDi-E--rwBujaNkTejLNI6ap" frameborder="0" allowfullscreen></iframe>
<iframe width="560" height="315" src="https://www.youtube.com/embed/Cn91MrBbxqc?list=PLLBUgWXdTBDi-E--rwBujaNkTejLNI6ap" frameborder="0" gesture="media" allow="encrypted-media" allowfullscreen></iframe>
<iframe width="560" height="315" src="https://www.youtube.com/embed/cXHvC_FGx24" frameborder="0" allowfullscreen></iframe>
<iframe width="560" height="315" src="https://www.youtube.com/embed/cXHvC_FGx24" frameborder="0" gesture="media" allow="encrypted-media" allowfullscreen></iframe>
- Initialize Model
- help(m)
- define parameter
eq = m.Param(value=40)
- initialize variables
x1,x2,x3,x4 = [m.Var() for i in range(4)]
- initial values
x1.value = 1 x2.value = 5 x3.value = 5 x4.value = 1
- lower bounds
x1.lower = 1 x2.lower = 1 x3.lower = 1 x4.lower = 1
- upper bounds
x1.upper = 5 x2.upper = 5 x3.upper = 5 x4.upper = 5
- Equations
x = m.Array(m.Var,4,value=1,lb=1,ub=5) x1,x2,x3,x4 = x
- change initial values
x2.value = 5; x3.value = 5
m.Equation(x1**2+x2**2+x3**2+x4**2==eq)
- Objective
m.Obj(x1*x4*(x1+x2+x3)+x3)
- Set global options
m.options.IMODE = 3 #steady state optimization
- Solve simulation
m.Equation(x1**2+x2**2+x3**2+x4**2==40) m.Minimize(x1*x4*(x1+x2+x3)+x3)
- Results
print('') print('Results') print('x1: ' + str(x1.value)) print('x2: ' + str(x2.value)) print('x3: ' + str(x3.value)) print('x4: ' + str(x4.value))
print('x: ', x) print('Objective: ',m.options.OBJFCNVAL)
(:html:)
<div id="disqus_thread"></div> <script type="text/javascript"> /* * * CONFIGURATION VARIABLES: EDIT BEFORE PASTING INTO YOUR WEBPAGE * * */ var disqus_shortname = 'apmonitor'; // required: replace example with your forum shortname /* * * DON'T EDIT BELOW THIS LINE * * */ (function() { var dsq = document.createElement('script'); dsq.type = 'text/javascript'; dsq.async = true; dsq.src = 'https://' + disqus_shortname + '.disqus.com/embed.js'; (document.getElementsByTagName('head')[0] || document.getElementsByTagName('body')[0]).appendChild(dsq); })(); </script> <noscript>Please enable JavaScript to view the <a href="https://disqus.com/?ref_noscript">comments powered by Disqus.</a></noscript> <a href="https://disqus.com" class="dsq-brlink">comments powered by <span class="logo-disqus">Disqus</span></a>
(:htmlend:)
x1.lb = 1 x2.lb = 1 x3.lb = 1 x4.lb = 1
x1.lower = 1 x2.lower = 1 x3.lower = 1 x4.lower = 1
x1.ub = 5 x2.ub = 5 x3.ub = 5 x4.ub = 5
x1.upper = 5 x2.upper = 5 x3.upper = 5 x4.upper = 5
(:html:) <iframe width="560" height="315" src="https://www.youtube.com/embed/SH753YX2K1A" frameborder="0" gesture="media" allow="encrypted-media" allowfullscreen></iframe> (:htmlend:)
(:html:) <iframe width="560" height="315" src="https://www.youtube.com/embed/cXHvC_FGx24" frameborder="0" allowfullscreen></iframe> (:htmlend:)
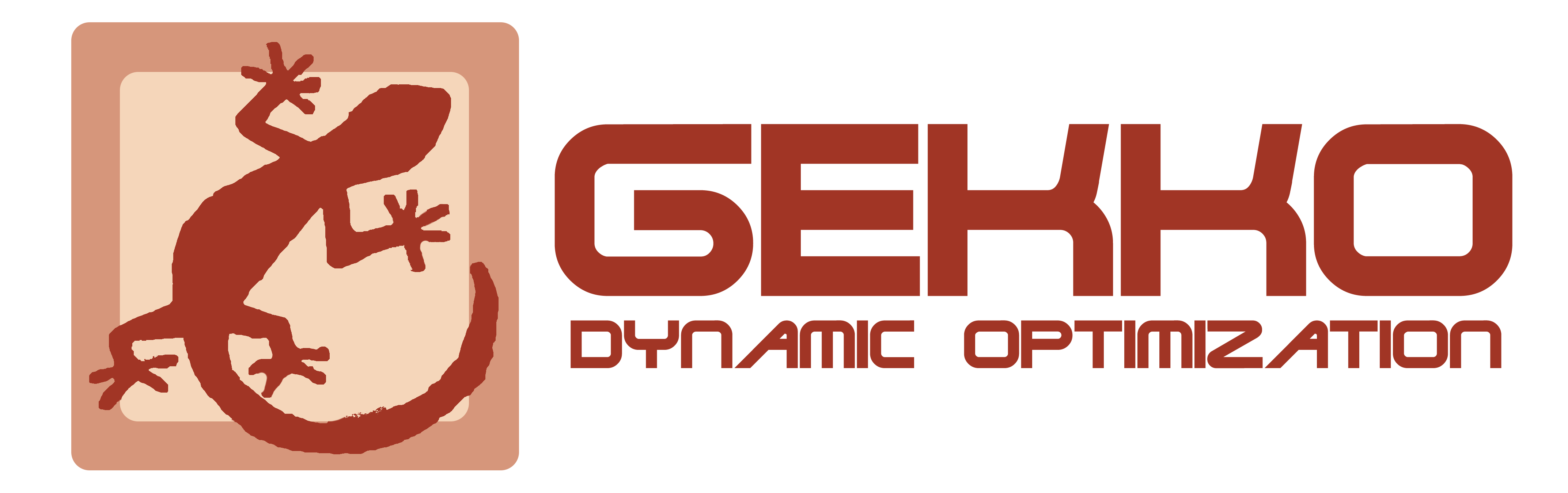
(:source lang=python:)
(:sourceend:)
(:toggle hide scipy button show="Show SciPy Solution":) (:div id=scipy:)
(:divend:)
Method #3: GEKKO Solution
(:html:) <iframe width="560" height="315" src="https://www.youtube.com/embed/cXHvC_FGx24" frameborder="0" allowfullscreen></iframe> (:htmlend:)
(:toggle hide gekko button show="Show GEKKO Solution":) (:div id=gekko:) from gekko import GEKKO import numpy as np
- Initialize Model
m = GEKKO()
- help(m)
- define parameter
eq = m.Param(value=40)
- initialize variables
x1,x2,x3,x4 = [m.Var() for i in range(4)]
- initial values
x1.value = 1 x2.value = 5 x3.value = 5 x4.value = 1
- lower bounds
x1.lb = 1 x2.lb = 1 x3.lb = 1 x4.lb = 1
- upper bounds
x1.ub = 5 x2.ub = 5 x3.ub = 5 x4.ub = 5
- Equations
m.Equation(x1*x2*x3*x4>=25) m.Equation(x1**2+x2**2+x3**2+x4**2==eq)
- Objective
m.Obj(x1*x4*(x1+x2+x3)+x3)
- Set global options
m.options.IMODE = 3 #steady state optimization
- Solve simulation
m.solve(remote=True)
- Results
print('') print('Results') print('x1: ' + str(x1.value)) print('x2: ' + str(x2.value)) print('x3: ' + str(x3.value)) print('x4: ' + str(x4.value)) (:divend:)
print('Initial SSE Objective: ' + str(objective(x0)))
print('Initial Objective: ' + str(objective(x0)))
print('Final SSE Objective: ' + str(objective(x)))
print('Final Objective: ' + str(objective(x)))
(:html:) <iframe width="560" height="315" src="https://www.youtube.com/embed/cXHvC_FGx24" frameborder="0" allowfullscreen></iframe> (:htmlend:)
Method #1: APM Python
Method #2: SciPy Optimize Minimize
(:source lang=python:) import numpy as np from scipy.optimize import minimize
def objective(x):
return x[0]*x[3]*(x[0]+x[1]+x[2])+x[2]
def constraint1(x):
return x[0]*x[1]*x[2]*x[3]-25.0
def constraint2(x):
sum_eq = 40.0 for i in range(4): sum_eq = sum_eq - x[i]**2 return sum_eq
- initial guesses
n = 4 x0 = np.zeros(n) x0[0] = 1.0 x0[1] = 5.0 x0[2] = 5.0 x0[3] = 1.0
- show initial objective
print('Initial SSE Objective: ' + str(objective(x0)))
- optimize
b = (1.0,5.0) bnds = (b, b, b, b) con1 = {'type': 'ineq', 'fun': constraint1} con2 = {'type': 'eq', 'fun': constraint2} cons = ([con1,con2]) solution = minimize(objective,x0,method='SLSQP', bounds=bnds,constraints=cons) x = solution.x
- show final objective
print('Final SSE Objective: ' + str(objective(x)))
- print solution
print('Solution') print('x1 = ' + str(x[0])) print('x2 = ' + str(x[1])) print('x3 = ' + str(x[2])) print('x4 = ' + str(x[3])) (:sourceend:)
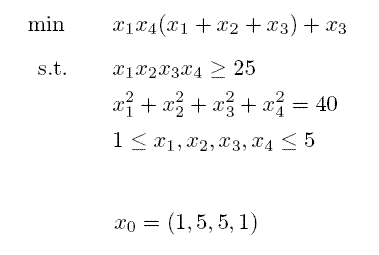
$$\min x_1 x_4 \left(x_1 + x_2 + x_3\right) + x_3$$ $$\mathrm{s.t.} \quad x_1 x_2 x_3 x_4 \ge 25$$ $$x_1^2 + x_2^2 + x_3^2 + x_4^2 = 40$$ $$1\le x_1, x_2, x_3, x_4 \le 5$$ $$x_0 = (1,5,5,1)$$
<iframe width="560" height="315" src="//www.youtube.com/embed/BZmSH8Y8_fc?list=PLLBUgWXdTBDi-E--rwBujaNkTejLNI6ap" frameborder="0" allowfullscreen></iframe>
<iframe width="560" height="315" src="//www.youtube.com/embed/Cn91MrBbxqc?list=PLLBUgWXdTBDi-E--rwBujaNkTejLNI6ap" frameborder="0" allowfullscreen></iframe>
Mathematical optimization problems may include equality constraints (e.g. =), inequality constraints (e.g. <, <=, >, >=), objective functions, algebraic equations, differential equations, continuous variables, discrete or integer variables, etc. One example of an optimization problem from a benchmark test set is the Hock Schittkowski problem #71.
Mathematical optimization problems may include equality constraints (e.g. =), inequality constraints (e.g. <, <=, >, >=), objective functions, algebraic equations, differential equations, continuous variables, discrete or integer variables, etc. One example of an optimization problem from a benchmark test set is the Hock Schittkowski problem #71.
(:title Optimization with Python:) (:keywords Optimization, Nonlinear Programming, Python, nonlinear, optimization, engineering optimization, university course:) (:description Optimization with Python - Problem-Solving Techniques for Chemical Engineers at Brigham Young University:)
Optimization deals with selecting the best option among a number of possible choices that are feasible or don't violate constraints. Python can be used to optimize parameters in a model to best fit data, increase profitability of a potential engineering design, or meet some other type of objective that can be described mathematically with variables and equations.
Mathematical optimization problems may include equality constraints (e.g. =), inequality constraints (e.g. <, <=, >, >=), objective functions, algebraic equations, differential equations, continuous variables, discrete or integer variables, etc. One example of an optimization problem from a benchmark test set is the Hock Schittkowski problem #71.
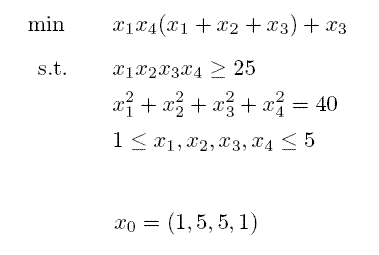
This problem has a nonlinear objective that the optimizer attempts to minimize. The variable values at the optimal solution are subject to (s.t.) both equality (=40) and inequality (>25) constraints. The product of the four variables must be greater than 25 while the sum of squares of the variables must also equal 40. In addition, all variables must be between 1 and 5 and the initial guess is x1 = 1, x2 = 5, x3 = 5, and x4 = 1.
(:html:) <iframe width="560" height="315" src="//www.youtube.com/embed/BZmSH8Y8_fc?list=PLLBUgWXdTBDi-E--rwBujaNkTejLNI6ap" frameborder="0" allowfullscreen></iframe> (:htmlend:)
This tutorial can also be completed with nonlinear programming optimizers that are available with the Excel Solver and MATLAB Optimization Toolbox. Click on the appropriate link for additional information and source code.
(:html:)
<div id="disqus_thread"></div> <script type="text/javascript"> /* * * CONFIGURATION VARIABLES: EDIT BEFORE PASTING INTO YOUR WEBPAGE * * */ var disqus_shortname = 'apmonitor'; // required: replace example with your forum shortname /* * * DON'T EDIT BELOW THIS LINE * * */ (function() { var dsq = document.createElement('script'); dsq.type = 'text/javascript'; dsq.async = true; dsq.src = 'https://' + disqus_shortname + '.disqus.com/embed.js'; (document.getElementsByTagName('head')[0] || document.getElementsByTagName('body')[0]).appendChild(dsq); })(); </script> <noscript>Please enable JavaScript to view the <a href="https://disqus.com/?ref_noscript">comments powered by Disqus.</a></noscript> <a href="https://disqus.com" class="dsq-brlink">comments powered by <span class="logo-disqus">Disqus</span></a>
(:htmlend:)